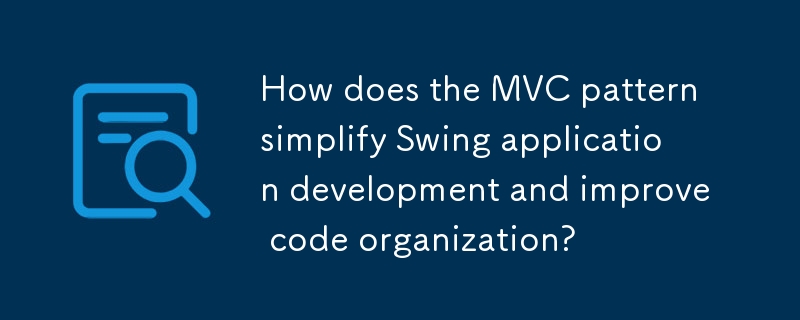
MVC Pattern in Swing
The Model-View-Controller (MVC) pattern is a design pattern commonly used in GUI programming to separate the user interface (the view) from the underlying business logic (the model) and the control logic (the controller). In the context of Swing, the MVC pattern helps in managing the complexity of GUI applications by separating these three concerns.
Understanding the MVC Pattern in Swing
Consider an application with a JFrame containing a table, text fields, and buttons. Typically, the view component (JFrame) would handle all the actions related to the UI elements, including clearing fields, validating input, locking fields, and handling button actions. However, this approach blurs the line between the controller and view.
To implement the MVC pattern correctly, we should separate the following responsibilities:
-
Model: Represents the underlying data and business logic.
-
View: Displays the data and UI elements to the user.
-
Controller: Handles user interactions, validates input, and updates the model.
In this scenario, the JTable would be the view component that displays the data from the underlying model (DataModel). The JFrame acts as a container or "frame" for the UI elements but should not be responsible for the controller-related actions.
Implementation of MVC in Swing
-
Use a Controller Class: Create a separate class that handles the controller responsibilities. This class should receive events from the view (e.g., button clicks) and update the model accordingly.
-
Define a View Interface: Create an interface that defines the methods that the view must implement. This interface should include methods for setting data, displaying the view, and handling user interactions.
-
Implement the View: Create a class that implements the view interface. This class should be responsible for displaying the GUI components and delegating user interactions to the controller.
-
Connect the Controller and View: Establish a relationship between the controller and the view. The view should hold a reference to the controller and delegate events to the appropriate methods in the controller.
-
Update the Model: When the controller receives an event, it updates the model accordingly. The model then notifies any registered observers (in this case, the view) about the changes.
-
React to Model Changes: The view observes the model for changes. When the model updates, the view reflects the changes in its display.
Additional Considerations
-
SwingWorker and Event Dispatch Thread: Swing applications use a single-threaded event dispatch thread to process GUI events. Resource-intensive tasks should be handled in a separate thread using SwingWorker.
-
Composite Design Patterns: MVC is often combined with other design patterns, such as Observer (for model-to-view updates) and Strategy (for configurable controllers).
-
Event Handling: User interactions with UI elements are delegated to the view. The view detects events and notifies the controller, which then takes appropriate actions.
The above is the detailed content of How does the MVC pattern simplify Swing application development and improve code organization?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn