


How Does JavaScript's `sort()` Method Use a Callback Function for Numerical Sorting?
How Javascript's Sort() Method Utilizes a Callback Function for Numerical Sorting
In Javascript, the sort() method enables arrays to be sorted in a customized manner. Understanding the intricacies of this method can be challenging, particularly for beginners.
How the Sort() Method Utilizes a Callback Function
The sort() method accepts a callback function as an argument. This function is invoked multiple times during the sorting process, with two numbers passed into it each time. These numbers represent the current elements being compared. The function's return value determines how these elements are ordered in the final sorted array.
Example:
Consider the following code:
var array=[25, 8, 7, 41] array.sort(function(a,b){ return a - b })
In this example, the sort() method is used to arrange the array array in ascending numerical order. The callback function is defined as follows:
function(a,b){ return a - b }
This callback function takes two parameters, a and b, representing the two elements being compared. It computes their difference (a - b).
Sorting Criteria
The result of the callback function's computation determines the sorting order. If the result is:
- Greater than 0: The element represented by a precedes the element represented by b in the sorted array.
- Equal to 0: The order of the elements remains unchanged.
- Less than 0: The element represented by b precedes the element represented by a in the sorted array.
Sorting Process
The sort() method iterates through the array, invoking the callback function for each pair of elements. Based on the function's output, it adjusts the order of the elements until the entire array is sorted.
Log of Callback Invocations (Example)
If we modified the callback function as follows:
function(a,b){ console.log(`comparing ${a},${b}`); return a > b ? 1 : a === b ? 0 : -1; }
We would obtain the following log of callback invocations:
comparing 25,8 comparing 25,7 comparing 8,7 comparing 25,41
This demonstrates that the callback function is indeed invoked multiple times, with the pairs of elements changing with each iteration.
In summary, Javascript's sort() method utilizes a callback function to determine the sorting order of array elements by comparing them in pairs. This callback function is invoked multiple times during the sorting process, and its output determines the final sorted array.
The above is the detailed content of How Does JavaScript's `sort()` Method Use a Callback Function for Numerical Sorting?. For more information, please follow other related articles on the PHP Chinese website!
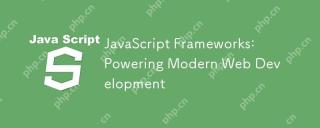
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
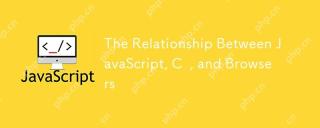
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
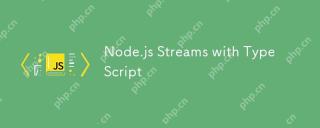
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
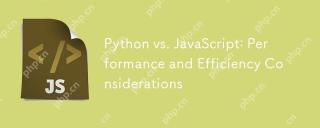
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
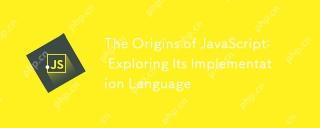
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
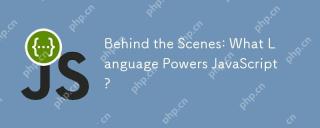
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
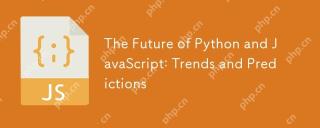
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
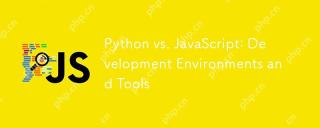
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version
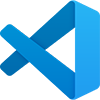
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
