How to Transition from MySQL Functions to PDO
Deprecated MySQL Functions
Modern PHP versions deprecate the MySQL functions due to their limitations and security concerns. Instead, developers should use the PDO or MySQLi extensions for improved database connectivity and security.
Introduction to PDO
PDO stands for PHP Data Objects and provides a consistent API for connecting to different database systems, including MySQL and MSSQL. Unlike MySQL functions, PDO uses a unified interface and offers increased security through prepared statements.
Connecting to Databases
MySQL Connection:
$dsn = 'mysql:dbname=databasename;host=127.0.0.1'; $user = 'dbuser'; $password = 'dbpass'; $dbh = new PDO($dsn, $user, $password);
MSSQL Connection:
$dsn = 'sqlsrv:Server=127.0.0.1;Database=databasename'; $user = 'dbuser'; $password = 'dbpass'; $dbh = new PDO($dsn, $user, $password);
Executing Queries
PDO uses prepared statements instead of plain SQL strings to prevent SQL injection vulnerabilities. Prepared statements are constructed with placeholders that are later bound to variables.
Named Placeholders:
$SQL = 'SELECT ID, EMAIL FROM users WHERE user = :username'; $queryArguments = array(':username' => $username); $result = $dbh->prepare($SQL); $result->execute($queryArguments);
Indexed Placeholders:
$SQL = 'SELECT ID, EMAIL FROM users WHERE user = ?'; $bindParamResults = array($username); $result = $dbh->prepare($SQL); $result->bindParam(1, $bindParamResults[0]); $result->execute();
Fetching Results
Results can be fetched using various methods, such as fetch() and fetchAll().
$row = $result->fetch(PDO::FETCH_ASSOC); // Returns an associative array $allRows = $result->fetchAll(PDO::FETCH_ASSOC); // Returns an array of associative arrays
Sample PDO Class
class PDOC { public function __construct($dsn, $user, $password) { $this->dbh = new PDO($dsn, $user, $password); } public function query($sql, $params = array()) { $stmt = $this->dbh->prepare($sql); $stmt->execute($params); return $stmt; } } $pdod = new PDOC('mysql:dbname=db;host=localhost', 'root', ''); $query = $pdod->query('SELECT * From table WHERE id = ?', array(2));
The above is the detailed content of How to Transition from Deprecated MySQL Functions to PDO in PHP?. For more information, please follow other related articles on the PHP Chinese website!
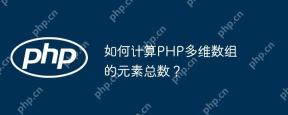
Calculating the total number of elements in a PHP multidimensional array can be done using recursive or iterative methods. 1. The recursive method counts by traversing the array and recursively processing nested arrays. 2. The iterative method uses the stack to simulate recursion to avoid depth problems. 3. The array_walk_recursive function can also be implemented, but it requires manual counting.
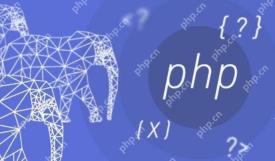
In PHP, the characteristic of a do-while loop is to ensure that the loop body is executed at least once, and then decide whether to continue the loop based on the conditions. 1) It executes the loop body before conditional checking, suitable for scenarios where operations need to be performed at least once, such as user input verification and menu systems. 2) However, the syntax of the do-while loop can cause confusion among newbies and may add unnecessary performance overhead.
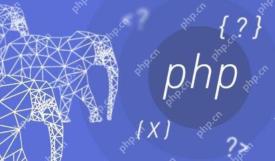
Efficient hashing strings in PHP can use the following methods: 1. Use the md5 function for fast hashing, but is not suitable for password storage. 2. Use the sha256 function to improve security. 3. Use the password_hash function to process passwords to provide the highest security and convenience.
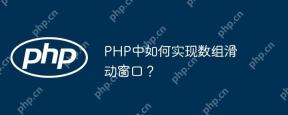
Implementing an array sliding window in PHP can be done by functions slideWindow and slideWindowAverage. 1. Use the slideWindow function to split an array into a fixed-size subarray. 2. Use the slideWindowAverage function to calculate the average value in each window. 3. For real-time data streams, asynchronous processing and outlier detection can be used using ReactPHP.
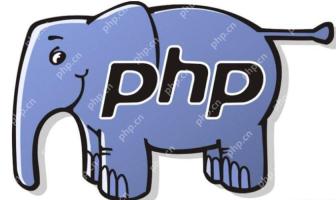
The __clone method in PHP is used to perform custom operations when object cloning. When cloning an object using the clone keyword, if the object has a __clone method, the method will be automatically called, allowing customized processing during the cloning process, such as resetting the reference type attribute to ensure the independence of the cloned object.
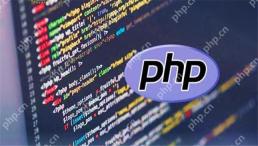
In PHP, goto statements are used to unconditionally jump to specific tags in the program. 1) It can simplify the processing of complex nested loops or conditional statements, but 2) Using goto may make the code difficult to understand and maintain, and 3) It is recommended to give priority to the use of structured control statements. Overall, goto should be used with caution and best practices are followed to ensure the readability and maintainability of the code.
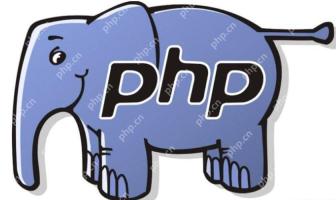
In PHP, data statistics can be achieved by using built-in functions, custom functions, and third-party libraries. 1) Use built-in functions such as array_sum() and count() to perform basic statistics. 2) Write custom functions to calculate complex statistics such as medians. 3) Use the PHP-ML library to perform advanced statistical analysis. Through these methods, data statistics can be performed efficiently.
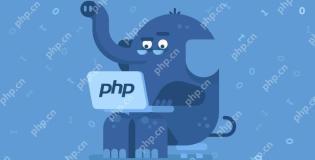
Yes, anonymous functions in PHP refer to functions without names. They can be passed as parameters to other functions and as return values of functions, making the code more flexible and efficient. When using anonymous functions, you need to pay attention to scope and performance issues.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
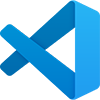
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
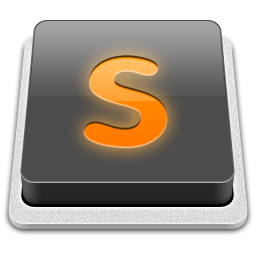
SublimeText3 Mac version
God-level code editing software (SublimeText3)
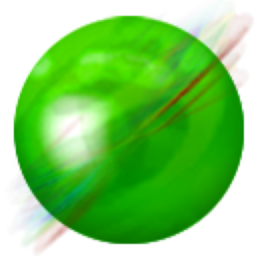
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
