Print Hierarchical Binary Tree in Java
To print a binary tree, showing its hierarchical structure in a text representation, you can utilize the Builder pattern. This pattern allows you to construct a tree-like output incrementally.
One approach is to create a custom TreeNode class that represents each node in the tree:
public class TreeNode<a extends comparable> { TreeNode</a><a> left, right; A data; }</a>
To print the tree, you can use a recursive helper function that builds up a string representation of the tree:
public String toString() { StringBuilder builder = new StringBuilder(); print(builder, "", ""); return builder.toString(); } private void print(StringBuilder builder, String prefix, String childrenPrefix) { builder.append(prefix).append(data).append('\n'); if (left != null) { left.print(builder, childrenPrefix + "├── ", childrenPrefix + "│ "); } if (right != null) { right.print(builder, childrenPrefix + "└── ", childrenPrefix + " "); } }
Here, prefix represents the prefix for the current node, and childrenPrefix represents the prefix for its children. The function recursively prints each child with an appropriate prefix indentation.
By calling toString() on the root node, you can obtain a hierarchical representation of the entire tree:
TreeNode<integer> root = new TreeNode(4); root.left = new TreeNode(2); root.right = new TreeNode(5); System.out.println(root.toString());</integer>
This will output:
4 ├── 2 └── 5
The above is the detailed content of How to Print a Binary Tree in Hierarchical Form in Java?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
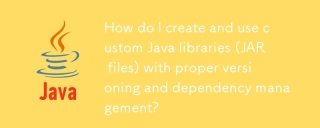
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
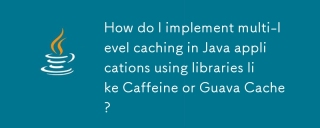
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
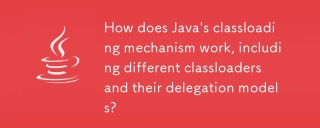
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
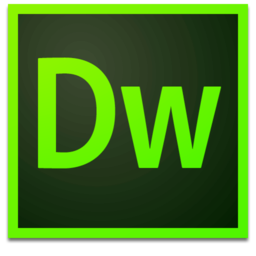
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.