Converting a String Representing Time to time_t in C
When working with time in C , you may encounter scenarios where you have a string that represents a specific time and need to convert it into the portable time_t data type. The time_t type represents time as the number of seconds elapsed since January 1, 1970, 00:00:00 UTC.
Conversion to time_t
In C 11 and later, the std::get_time function can be used to parse a string into a std::tm struct, which can then be converted to time_t using mktime:
#include <iostream> #include <sstream> #include <time.h> int main() { std::string time_details = "16:35:12"; std::tm tm; std::istringstream ss(time_details); ss >> std::get_time(&tm, "%H:%M:%S"); std::time_t time = mktime(&tm); // Print the converted time_t std::cout <p><strong>Comparing Two Times</strong></p> <p>Once you have multiple times in the <strong>time_t</strong> format, you can compare them to determine which is earlier.</p> <pre class="brush:php;toolbar:false">#include <iostream> #include <time.h> int main() { std::string curr_time = "18:35:21"; // Current time std::string user_time = "22:45:31"; // User's time std::tm curr_tm, user_tm; std::istringstream ss; // Parse both times ss.str(curr_time); ss >> std::get_time(&curr_tm, "%H:%M:%S"); ss.clear(); ss.str(user_time); ss >> std::get_time(&user_tm, "%H:%M:%S"); // Compare the time_t values std::time_t curr_secs = mktime(&curr_tm); std::time_t user_secs = mktime(&user_tm); if (curr_secs </time.h></iostream>
The above is the detailed content of How to Convert a String Representing Time to a `time_t` in C ?. For more information, please follow other related articles on the PHP Chinese website!
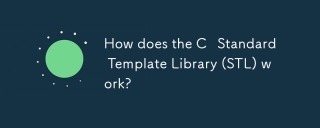
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
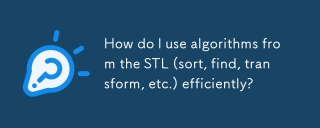
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
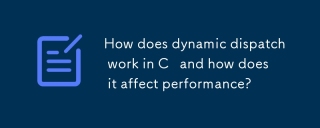
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
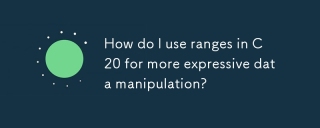
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
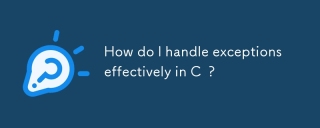
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
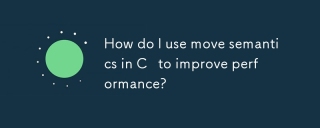
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
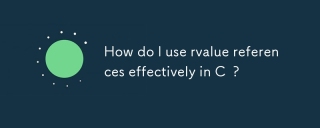
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
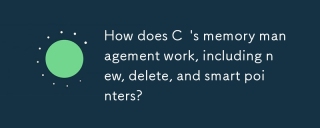
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
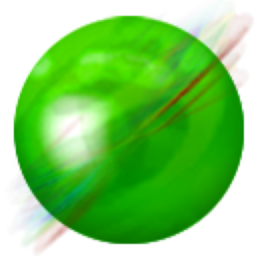
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
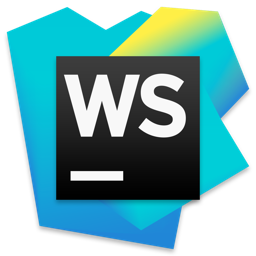
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
