How to Measure CPU and Wall Clock Time in Linux and Windows
Measuring CPU and Wall Clock Time
To effectively analyze and optimize the performance of your code, accurately measuring both CPU time and wall clock time is essential. Let's delve into how this can be achieved on both Linux and Windows platforms.
CPU Time vs. Wall Clock Time
- CPU Time: Represents the amount of time the CPU spends executing a particular function or code block. It excludes time spent on other tasks, such as disk or network I/O.
- Wall Clock Time: Measures the total time it takes to execute a function or code block, including time spent on all tasks, including CPU processing, I/O, and thread switching.
How to Measure CPU Time
- Linux: Use clock() function, which returns the amount of CPU time spent by the current process in seconds.
- Windows: Use GetProcessTimes() function, which provides information about various process times, including CPU user time.
How to Measure Wall Clock Time
- Linux: Use gettimeofday() function, which returns the current time with microsecond precision.
- Windows: Use QueryPerformanceCounter() function, which provides high-precision timing information.
Platform Independence
The methods described above are not inherently architecture-independent. Performance counters, clock functions, and time measurement mechanisms can vary across different CPU architectures, such as x86 and x86_64. However, the general principles of measuring CPU time and wall clock time remain the same.
Code Example
Here's an example code snippet that demonstrates how to measure both CPU and wall clock time in C :
#include <iostream> #include <chrono> using namespace std; int main() { // Declare variables to measure time auto startCPU = chrono::high_resolution_clock::now(); auto startWall = chrono::system_clock::now(); // Perform some CPU-intensive computations here // Stop time measurements auto endCPU = chrono::high_resolution_clock::now(); auto endWall = chrono::system_clock::now(); // Calculate CPU time chrono::duration<double> cpuTime = endCPU - startCPU; // Calculate wall clock time chrono::duration<double> wallClockTime = endWall - startWall; cout <p>By using the above code snippet, you can accurately measure and analyze the performance of your code in terms of both CPU time and wall clock time.</p></double></double></chrono></iostream>
The above is the detailed content of How to measure CPU and Wall Clock Time in Linux and Windows?. For more information, please follow other related articles on the PHP Chinese website!
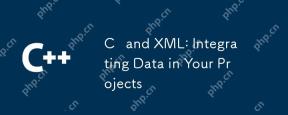
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
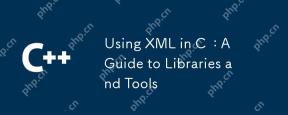
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
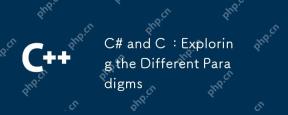
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
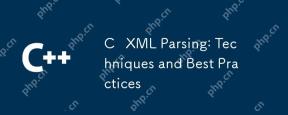
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
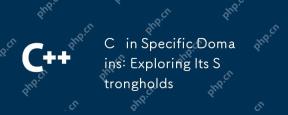
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
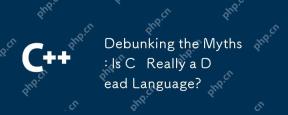
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
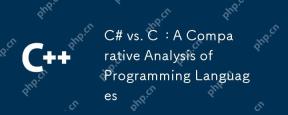
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
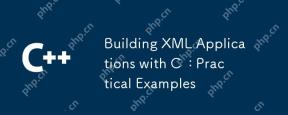
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
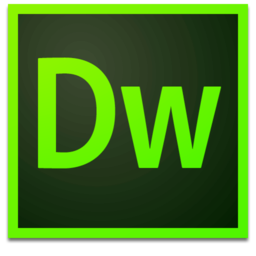
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!
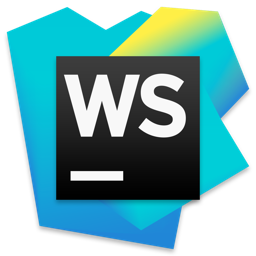
WebStorm Mac version
Useful JavaScript development tools
