Storing ECDSA Private Keys in Go
When working with ECDSA key pairs in Go, the need often arises to store the private key securely. This guide will delve into techniques for effectively storing private keys in files on the user's computer.
Encoding and Decoding Private Keys
Go does not provide a direct mechanism for marshaling private keys using the elliptic.Marshal method. Instead, a multi-step encoding process is required:
- Cryptographic Algorithm: Use the ECDSA algorithm to generate a key, such as ecdsa.GenerateKey(elliptic.P384(), rand.Reader).
- Standard Encoding: Convert the private key to x509 format using x509.MarshalECPrivateKey(privateKey).
- File Format: Encode the x509 structure to PEM format using pem.EncodeToMemory(block), where block represents the x509 structure.
Sample Code
The following Go code demonstrates the above process:
package main import ( "crypto/ecdsa" "crypto/elliptic" "crypto/rand" "crypto/x509" "encoding/pem" "fmt" "reflect" ) func encode(privateKey *ecdsa.PrivateKey, publicKey *ecdsa.PublicKey) (string, string) { x509Encoded, _ := x509.MarshalECPrivateKey(privateKey) pemEncoded := pem.EncodeToMemory(&pem.Block{Type: "PRIVATE KEY", Bytes: x509Encoded}) x509EncodedPub, _ := x509.MarshalPKIXPublicKey(publicKey) pemEncodedPub := pem.EncodeToMemory(&pem.Block{Type: "PUBLIC KEY", Bytes: x509EncodedPub}) return string(pemEncoded), string(pemEncodedPub) } func decode(pemEncoded string, pemEncodedPub string) (*ecdsa.PrivateKey, *ecdsa.PublicKey) { block, _ := pem.Decode([]byte(pemEncoded)) x509Encoded := block.Bytes privateKey, _ := x509.ParseECPrivateKey(x509Encoded) blockPub, _ := pem.Decode([]byte(pemEncodedPub)) x509EncodedPub := blockPub.Bytes genericPublicKey, _ := x509.ParsePKIXPublicKey(x509EncodedPub) publicKey := genericPublicKey.(*ecdsa.PublicKey) return privateKey, publicKey } func main() { privateKey, _ := ecdsa.GenerateKey(elliptic.P384(), rand.Reader) publicKey := &privateKey.PublicKey encPriv, encPub := encode(privateKey, publicKey) fmt.Println(encPriv) fmt.Println(encPub) priv2, pub2 := decode(encPriv, encPub) if !reflect.DeepEqual(privateKey, priv2) { fmt.Println("Private keys do not match.") } if !reflect.DeepEqual(publicKey, pub2) { fmt.Println("Public keys do not match.") } }
By storing the PEM-encoded private key as a file, it can be retrieved and decoded later as needed. Remember to employ appropriate security measures to protect the file from unauthorized access or exposure.
The above is the detailed content of How to Store ECDSA Private Keys Securely in Go?. For more information, please follow other related articles on the PHP Chinese website!
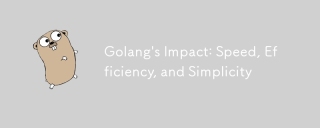
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
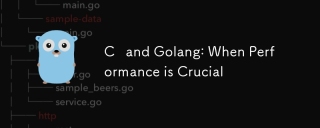
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
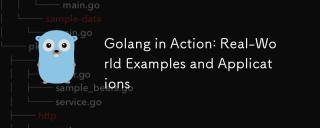
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
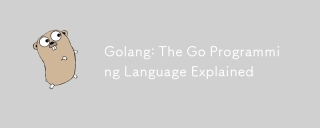
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
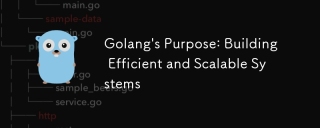
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
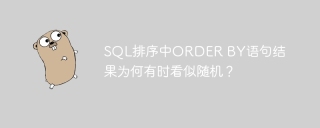
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
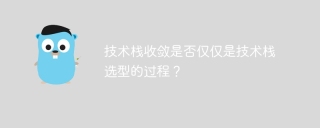
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
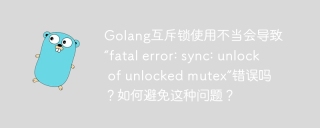
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
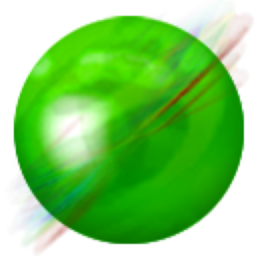
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
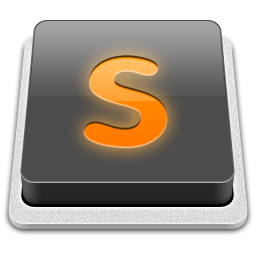
SublimeText3 Mac version
God-level code editing software (SublimeText3)