


Constructing DataFrames from Dictionaries with Uneven Array Lengths
Handling dictionaries with arrays of unequal lengths in Pandas requires a tailored approach. When attempting to create a DataFrame with each column representing an array within the dictionary, one may encounter the ValueError: "arrays must all be the same length."
Leveraging Series Objects
To circumvent this issue, we leverage Pandas' Series objects which can hold arrays of varying lengths. By converting each dictionary value into a Series, we can effectively store the arrays regardless of their lengths. The following code snippet demonstrates this approach:
import pandas as pd import numpy as np # Sample data generated via a reproducible seed np.random.seed(2023) data = {k: np.random.randn(v) for k, v in zip("ABCDEF", [10, 12, 15, 17, 20, 23])} # Convert dictionary values to Series objects series_dict = {k: pd.Series(v) for k, v in data.items()} # Create DataFrame using these Series objects df = pd.DataFrame(series_dict)
Preserving Missing Values
When working with arrays of varying lengths, it's common to encounter missing values where shorter arrays cannot fill the remaining cells. By default, Pandas fills these gaps with NaN (Not a Number) values. This behavior preserves the original data while providing a consistent structure for analysis.
Configuring Missing Value Handling
If desired, you can customize the handling of missing values by using the missing_values parameter in the DataFrame() constructor. For example, to replace missing values with zeros instead of NaN, you would specify missing_values=0 as shown below:
df = pd.DataFrame(series_dict, missing_values=0)
Example Output
The following output illustrates a DataFrame created using the approach outlined above:
print(df)
A B C D E F 0 0.711674 -1.076522 -1.502178 -1.519748 0.340619 0.051132 1 -0.324485 -0.325682 -1.379593 2.097329 -1.253501 -0.238061 2 -1.001871 -1.035498 -0.204455 0.892562 0.370788 -0.208009 3 0.236251 -0.426320 0.642125 1.596488 0.455254 0.401304 4 -0.102160 -1.029361 -0.181176 -0.638762 -2.283720 0.183169 ... ... ... ... ... ... ... 18 NaN NaN NaN NaN NaN NaN 19 NaN NaN NaN NaN NaN NaN 20 NaN NaN NaN NaN NaN NaN 21 NaN NaN NaN NaN NaN NaN 22 NaN NaN NaN NaN NaN NaN 23 rows × 6 columns
As you can observe, the shorter arrays result in NaN values in the corresponding cells, providing a comprehensive representation of your data while maintaining the desired tabular format.
The above is the detailed content of How to Construct Pandas DataFrames from Dictionaries with Uneven Array Lengths?. For more information, please follow other related articles on the PHP Chinese website!
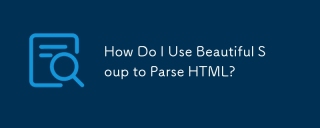
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
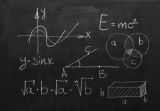
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
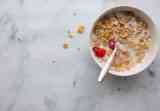
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
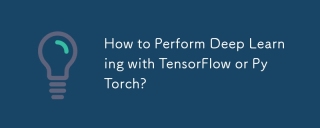
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
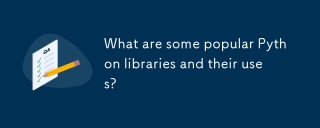
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
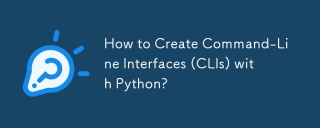
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
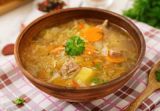
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
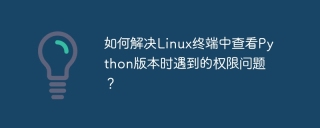
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
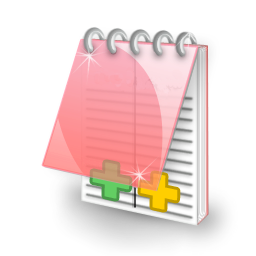
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
