Storing ECDSA Private Key in Go
When working with ECDSA key pairs in Go, the need arises to store the private key securely. While the elliptic.Marshal method provides encoding for the public key, there's no equivalent for the private key. This article explores how to save and load private keys in Go.
Encoding and Decoding
To store the private key, it's necessary to adopt a multi-step approach involving ECDSA key encryption, standard encoding, and a file format. The common combination involves using the ECDSA algorithm for key generation, X.509 for encoding, and the PEM (Privacy-Enhanced Mail) format for storage.
Code Example
The following code snippet demonstrates how to encode and decode ECDSA keys in Go:
import ( "crypto/ecdsa" "crypto/elliptic" "crypto/rand" "crypto/x509" "encoding/pem" "fmt" "reflect" ) func encode(privateKey *ecdsa.PrivateKey, publicKey *ecdsa.PublicKey) (string, string) { x509Encoded, _ := x509.MarshalECPrivateKey(privateKey) pemEncoded := pem.EncodeToMemory(&pem.Block{Type: "PRIVATE KEY", Bytes: x509Encoded}) x509EncodedPub, _ := x509.MarshalPKIXPublicKey(publicKey) pemEncodedPub := pem.EncodeToMemory(&pem.Block{Type: "PUBLIC KEY", Bytes: x509EncodedPub}) return string(pemEncoded), string(pemEncodedPub) } func decode(pemEncoded string, pemEncodedPub string) (*ecdsa.PrivateKey, *ecdsa.PublicKey) { block, _ := pem.Decode([]byte(pemEncoded)) x509Encoded := block.Bytes privateKey, _ := x509.ParseECPrivateKey(x509Encoded) blockPub, _ := pem.Decode([]byte(pemEncodedPub)) x509EncodedPub := blockPub.Bytes genericPublicKey, _ := x509.ParsePKIXPublicKey(x509EncodedPub) publicKey := genericPublicKey.(*ecdsa.PublicKey) return privateKey, publicKey } func test() { privateKey, _ := ecdsa.GenerateKey(elliptic.P384(), rand.Reader) publicKey := &privateKey.PublicKey encPriv, encPub := encode(privateKey, publicKey) fmt.Println(encPriv) fmt.Println(encPub) priv2, pub2 := decode(encPriv, encPub) if !reflect.DeepEqual(privateKey, priv2) { fmt.Println("Private keys do not match.") } if !reflect.DeepEqual(publicKey, pub2) { fmt.Println("Public keys do not match.") } }
In the test function:
- A new ECDSA private/public key pair is generated.
- The private and public keys are encoded and the encoded strings are printed.
- The encoded strings are decoded to recover the original keys.
- The recovered keys are compared to the original ones to verify if they match.
By utilizing the techniques outlined above, you can securely store and retrieve ECDSA private keys in Go, enabling the creation and management of digital signatures within your applications.
The above is the detailed content of How can I securely store and retrieve ECDSA private keys in Go?. For more information, please follow other related articles on the PHP Chinese website!
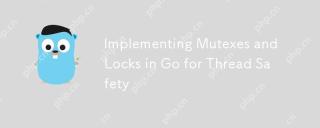
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
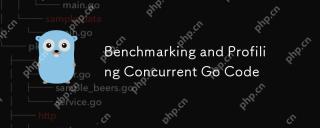
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
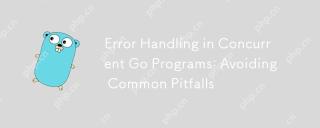
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.
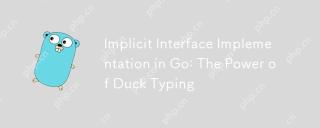
ImplicitinterfaceimplementationinGoembodiesducktypingbyallowingtypestosatisfyinterfaceswithoutexplicitdeclaration.1)Itpromotesflexibilityandmodularitybyfocusingonbehavior.2)Challengesincludeupdatingmethodsignaturesandtrackingimplementations.3)Toolsli
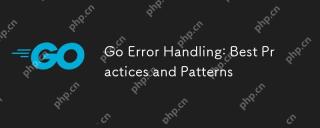
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
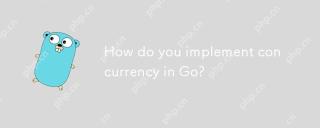
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
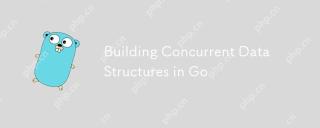
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
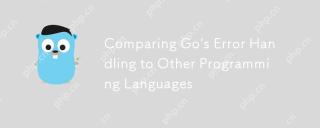
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
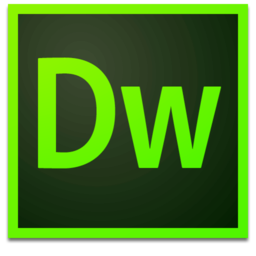
Dreamweaver Mac version
Visual web development tools
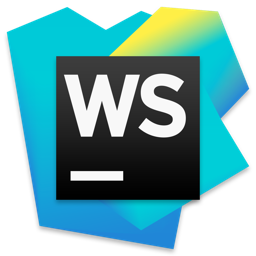
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
