


The Levenshtein distance, also known as the edit distance, is an essential metric for assessing the similarity between two strings. It counts the minimum number of operations necessary to transform one string into another. These operations include:
- Insert: Add a character.
- Delete: Delete a character.
- Substitution: Replace one character with another.
This concept is at the heart of many modern applications, such as spelling correction, fuzzy search, and DNA comparison.
The Mathematical Concept
The Levenshtein distance between two strings (A) and (B) of lengths (n) and (m), respectively, can be calculated using a dynamic approach. We define a matrix (D) of dimensions ((n 1) times (m 1)), where each (D[i][j]) represents the minimum cost to transform the (i) first characters of (A) into the (j) first characters of (B).
The recurrence formula is:
Implementation in Python
Here is a simple Python implementation to calculate the Levenshtein distance:
def levenshtein_distance(a, b): n, m = len(a), len(b) dp = [[0] * (m + 1) for _ in range(n + 1)] for i in range(n + 1): for j in range(m + 1): if i == 0: dp[i][j] = j elif j == 0: dp[i][j] = i elif a[i - 1] == b[j - 1]: dp[i][j] = dp[i - 1][j - 1] else: dp[i][j] = 1 + min(dp[i - 1][j], dp[i][j - 1], dp[i - 1][j - 1]) return dp[n][m] # Exemple d'utilisation print(levenshtein_distance("kitten", "sitting")) # Sortie : 3
Practical Applications
1. Spelling Correction
Spell checkers use Levenshtein to suggest close words in case of typos. For example, if you type helo, it might suggest hello or hero.
2. Fuzzy Search
In search engines, the Levenshtein distance allows you to obtain results even when the user makes typing errors.
3. DNA Comparison
In bioinformatics, this distance helps measure the similarity between two DNA sequences, each operation representing a possible mutation.
4. Authentication and Fraud Detection
Identity theft detection systems can compare user input with existing data, taking into account small textual differences.
Optimization: Levenshtein Distance with Reduced Memory
The classic algorithm uses a full matrix, which can be memory intensive. Fortunately, we can optimize using only two lines of memory, because each calculation ( D[i][j] ) depends only on ( D[i-1][j] ), ( D[i][j-1] ), and (D[i-1][j-1]).
def levenshtein_distance(a, b): n, m = len(a), len(b) dp = [[0] * (m + 1) for _ in range(n + 1)] for i in range(n + 1): for j in range(m + 1): if i == 0: dp[i][j] = j elif j == 0: dp[i][j] = i elif a[i - 1] == b[j - 1]: dp[i][j] = dp[i - 1][j - 1] else: dp[i][j] = 1 + min(dp[i - 1][j], dp[i][j - 1], dp[i - 1][j - 1]) return dp[n][m] # Exemple d'utilisation print(levenshtein_distance("kitten", "sitting")) # Sortie : 3
Conclusion
The Levenshtein distance is a powerful, versatile and widely used tool in many fields. Although it is simple to understand, its complex optimizations and applications demonstrate its value in modern systems.
Exploring further, we can also turn to variants like the Damerau-Levenshtein distance, which takes transpositions into account. You are now equipped to integrate this tool into your projects or simply impress your peers with your in-depth knowledge!
Do you have questions or ideas about the Levenshtein distance? Share them in the comments! ?
The above is the detailed content of Levenshtein Distance: The Ultimate Guide to Measuring Textual Similarity. For more information, please follow other related articles on the PHP Chinese website!
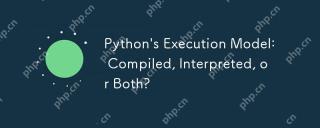
Pythonisbothcompiledandinterpreted.WhenyourunaPythonscript,itisfirstcompiledintobytecode,whichisthenexecutedbythePythonVirtualMachine(PVM).Thishybridapproachallowsforplatform-independentcodebutcanbeslowerthannativemachinecodeexecution.
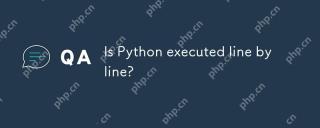
Python is not strictly line-by-line execution, but is optimized and conditional execution based on the interpreter mechanism. The interpreter converts the code to bytecode, executed by the PVM, and may precompile constant expressions or optimize loops. Understanding these mechanisms helps optimize code and improve efficiency.
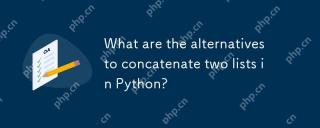
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
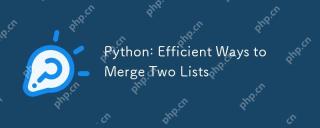
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
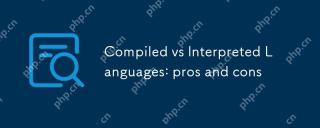
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
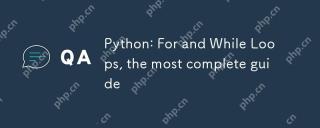
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
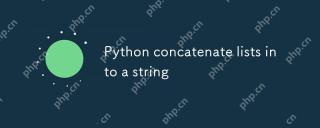
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
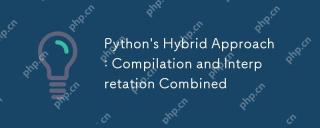
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
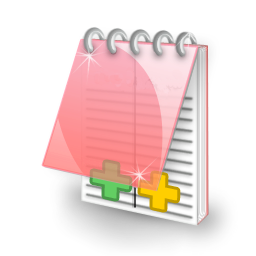
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
