


Create an Animated Sprite Using a Sequence of Images
In Python using Pygame, you can easily create animated sprites from a series of images:
Prerequisites:
- Gather your images as a sequence (e.g., explosion frames as separate PNGs).
- Ensure they have uniform dimensions.
Time-Dependent Animation:
- Load and Store Images: Create a list to hold the images and load them into it.
- Define Variables: Initialize an index to track the current image, a current time to measure time elapsed since the last image switch, and an animation time for switching images.
-
Main Loop Update:
- Increment the current time.
-
If the current time exceeds the animation time:
- Reset the current time to 0.
- Increment the index, and reset it if it reaches the number of images.
- Change the sprite's image to the new index.
Frame-Dependent Animation:
Similar to time-dependent animation, but instead of using time, increment the current frame count:
-
Main Loop Update:
- Increment the current frame.
-
If the current frame exceeds the animation frame count:
- Reset the current frame to 0.
- Increment the index, and reset it if it reaches the number of images.
- Change the sprite's image to the new index.
Working Example:
import pygame class AnimatedSprite(pygame.sprite.Sprite): def __init__(self, position, images): super().__init__() self.images = images self.index = 0 self.image = images[self.index] self.rect = self.image.get_rect(topleft=position) self.animation_time = 0.1 self.current_time = 0 def update(self, dt): self.current_time += dt if self.current_time >= self.animation_time: self.current_time = 0 self.index = (self.index + 1) % len(self.images) self.image = self.images[self.index]
Choosing Between Time-Dependent and Frame-Dependent:
- Time-Dependent: Ensures consistent animation speed regardless of frame rate, but may result in uneven spacing.
- Frame-Dependent: Can appear smoother with consistent frame rates but is affected by lagging.
The above is the detailed content of How to Create an Animated Sprite Using Image Sequences in Pygame?. For more information, please follow other related articles on the PHP Chinese website!
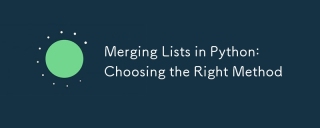
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
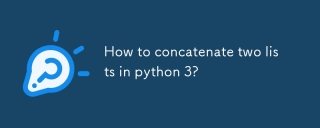
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
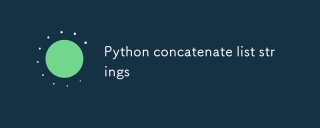
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
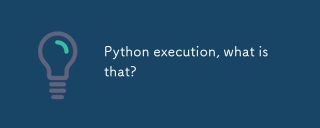
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
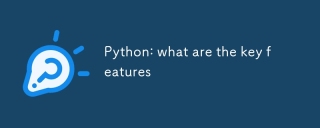
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
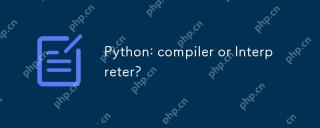
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
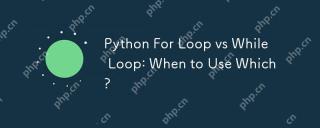
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
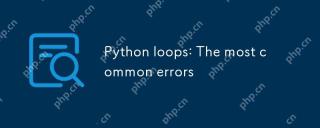
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use
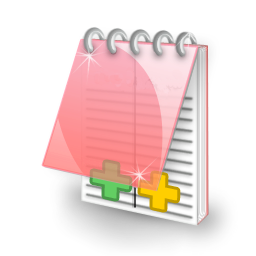
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor
