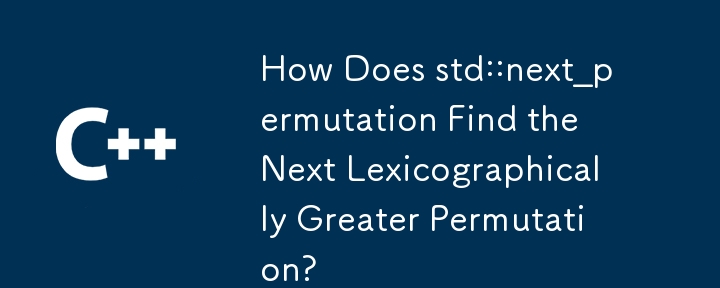
How std::next_permutation Works
std::next_permutation is a function in the C Standard Template Library (STL) that reorders a sequence into the next lexicographically greater permutation. To understand its implementation, it's helpful to visualize the sequence as a number where each element represents a digit.
Core Logic
The algorithm operates according to the following principles:
-
Find the Pivot: Starting from the end of the sequence, it locates the first element (i) that is less than the element to its right (j). This indicates that the digits to the right of i are in descending order.
-
Swap and Reverse: Once i is found, it searches from the end for the first element (k) that is greater than i. This element is swapped with i, placing it at the front. The remaining elements to the right of j (from j to end) are then reversed.
-
Increment the Pivot: If a pivot is found (i is not the beginning), the process repeats by decrementing i and j.
-
Reverse and Exit: If a pivot cannot be found (i is the beginning), the sequence is reversed and the function returns false, indicating that no more permutations are possible.
Variables in the Code
-
i: Represents the leftmost pivot element.
-
j: Represents the element to the right of i that is less than i.
-
k: Represents the element from the right that is greater than i and will be swapped with i.
Example
Consider the sequence: 1, 3, 2, 4.
-
Find the Pivot: i is initially set to 4, but since 4 is greater than or equal to 2, we move to i = 2. Since 2 is less than 4, i is the pivot.
-
Swap and Reverse: j is set to 3 and k is set to 1, which is the first element from the right that is greater than 2. 1 is swapped with 2, resulting in 1, 2, 3, 4. The remaining elements from j to end (2, 3, 4) are reversed, giving 1, 2, 4, 3.
-
Increment the Pivot: i is decremented to 1 (j is already set to 2). Since 1 is less than 2, the process repeats.
-
Find the Pivot: i is decremented to the first element (beginning), indicating that a pivot cannot be found.
-
Reverse and Exit: The sequence is reversed to its original state 1, 2, 3, 4 and the function returns false, indicating that no more permutations are possible.
The above is the detailed content of How Does std::next_permutation Find the Next Lexicographically Greater Permutation?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn