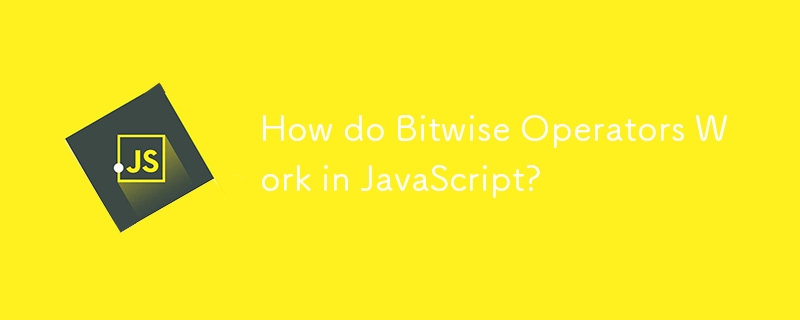
Understanding JavaScript Bitwise Operators
JavaScript offers a set of bitwise operators that manipulate individual bits within numbers. These operators enable efficient bit-level operations, allowing developers to perform tasks such as bit shifting, logical comparisons, and bit manipulation.
The bitwise operators in JavaScript include:
- x <<= y (x = x << y): Bitwise left shift. Shifts the bits of x left by y positions, effectively multiplying x by 2^y.
- x >>= y (x = x >> y): Bitwise right shift. Shifts the bits of x right by y positions, effectively dividing x by 2^y.
- x >>>= y (x = x >>> y): Unsigned bitwise right shift. Similar to >>=, but shifts a zero for the vacated bit positions.
- x &= y (x = x & y): Bitwise AND. Performs a logical AND operation on the bits of x and y, resulting in a value where a bit is set to 1 if it is 1 in both x and y.
- x ^= y (x = x ^ y): Bitwise XOR. Performs a logical XOR operation on the bits of x and y, resulting in a value where a bit is set to 1 if it is 1 in either x or y but not both.
- x |= y (x = x | y): Bitwise OR. Performs a logical OR operation on the bits of x and y, resulting in a value where a bit is set to 1 if it is 1 in either x or y.
These operators are powerful tools for performing bit-level manipulations in JavaScript programs, enabling developers to efficiently handle data at the bit level.
The above is the detailed content of How do Bitwise Operators Work in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn