


How to Customize Date Parsing for time.Time Fields in Go When Unmarshalling XML?
Custom XML Date Parsing in Go with time.Time Fields
Golang provides the flexibility to unmarshal XML data into custom structs, but it doesn't support specifying custom date formats for time.Time fields when unmarshaling XML. This can lead to parsing errors when the returned date format differs from the default Go format.
CustomUnmarshaler Solution
To overcome this limitation, you can create a custom struct with an anonymous time.Time field and implement your own UnmarshalXML method. This method will parse the date string using your custom format and set the time.Time field accordingly.
type customTime struct { time.Time } func (c *customTime) UnmarshalXML(d *xml.Decoder, start xml.StartElement) error { const shortForm = "20060102" // yyyymmdd date format var v string d.DecodeElement(&v, &start) parse, err := time.Parse(shortForm, v) if err != nil { return err } *c = customTime{parse} return nil }
Incorporating the Custom Struct
Update your Transaction struct to include the custom time field:
type Transaction struct { //... DateEntered customTime `xml:"enterdate"` //... }
Now, the XML unmarshalling will use the customTime struct and parse the date string according to your defined format.
Additional Notes
If the XML element uses an attribute for the date, you need to implement UnmarshalXMLAttr in the same manner. Refer to the example in the provided code snippet for guidance.
The above is the detailed content of How to Customize Date Parsing for time.Time Fields in Go When Unmarshalling XML?. For more information, please follow other related articles on the PHP Chinese website!
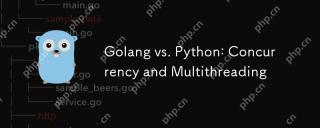
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
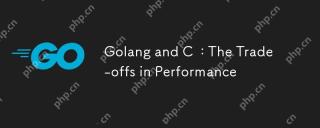
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
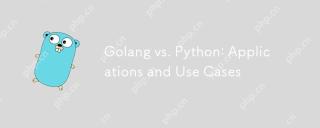
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
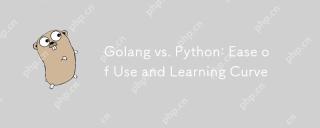
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
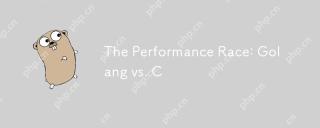
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
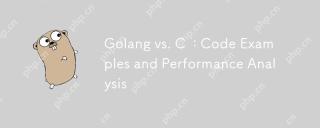
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
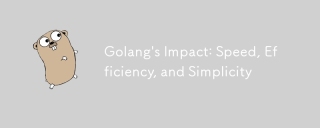
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
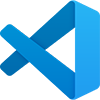
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
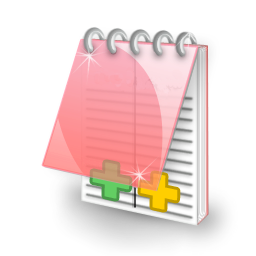
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function