Calling MySQL Stored Procedures with Both Input and Output Parameters in PHP
When working with MySQL stored procedures, it's often necessary to pass in and retrieve data from the database. This is where input and output parameters come into play. However, it can be challenging to use both input and output parameters, especially without using "INOUT" parameters.
Understanding the Procedure's Input and Output Parameters
Let's consider the example stored procedure in the MySQL database:
CREATE PROCEDURE `test_proc`( in input_param_1 int, in input_param_2 int, in input_param_3 int, out output_sum int, out output_product int, out output_average int )
This procedure expects three input parameters (input_param_1, input_param_2, input_param_3) and three output parameters (output_sum, output_product, output_average). The input parameters are used to calculate the output parameters.
Binding Input and Output Parameters Using MySQLi
To call this stored procedure from PHP using MySQLi, you'll need to bind both the input and output parameters. In the following code, we use the procedural interface to establish a connection, prepare the stored procedure call, bind the input parameters, execute the call, and retrieve the output parameters from user variables:
<?php $procInput1 = 123; $procInput2 = 456; $procInput3 = 789; $mysqli = mysqli_connect(...); $call = mysqli_prepare($mysqli, 'CALL test_proc(?, ?, ?, @sum, @product, @average)'); mysqli_stmt_bind_param($call, 'iii', $procInput1, $procInput2, $procInput3); mysqli_stmt_execute($call); $select = mysqli_query($mysqli, 'SELECT @sum, @product, @average'); $result = mysqli_fetch_assoc($select); $procOutput_sum = $result['@sum']; $procOutput_product = $result['@product']; $procOutput_average = $result['@average']; mysqli_stmt_close($call); mysqli_close($mysqli); ?>
In the above code, the mysqli_prepare function prepares the stored procedure call. The mysqli_stmt_bind_param function binds the input parameters to the call. The mysqli_stmt_execute function executes the call.
After executing the stored procedure, we have to retrieve the output parameters from the user variables using a separate SELECT statement. This is because MySQLi lacks native support for retrieving output parameters directly.
Conclusion
By understanding how to bind both input and output parameters in MySQL stored procedures using MySQLi, you can effectively interact with your database and retrieve the necessary data for your PHP applications.
The above is the detailed content of How to Retrieve Output Parameters from MySQL Stored Procedures in PHP?. For more information, please follow other related articles on the PHP Chinese website!
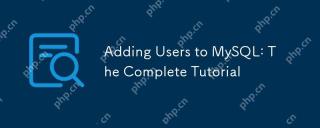
Mastering the method of adding MySQL users is crucial for database administrators and developers because it ensures the security and access control of the database. 1) Create a new user using the CREATEUSER command, 2) Assign permissions through the GRANT command, 3) Use FLUSHPRIVILEGES to ensure permissions take effect, 4) Regularly audit and clean user accounts to maintain performance and security.
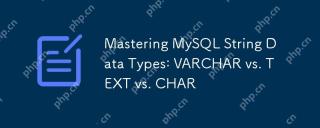
ChooseCHARforfixed-lengthdata,VARCHARforvariable-lengthdata,andTEXTforlargetextfields.1)CHARisefficientforconsistent-lengthdatalikecodes.2)VARCHARsuitsvariable-lengthdatalikenames,balancingflexibilityandperformance.3)TEXTisidealforlargetextslikeartic
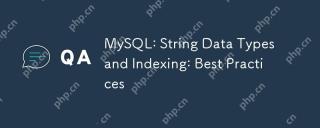
Best practices for handling string data types and indexes in MySQL include: 1) Selecting the appropriate string type, such as CHAR for fixed length, VARCHAR for variable length, and TEXT for large text; 2) Be cautious in indexing, avoid over-indexing, and create indexes for common queries; 3) Use prefix indexes and full-text indexes to optimize long string searches; 4) Regularly monitor and optimize indexes to keep indexes small and efficient. Through these methods, we can balance read and write performance and improve database efficiency.
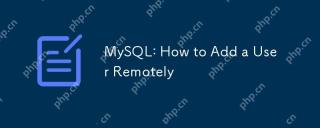
ToaddauserremotelytoMySQL,followthesesteps:1)ConnecttoMySQLasroot,2)Createanewuserwithremoteaccess,3)Grantnecessaryprivileges,and4)Flushprivileges.BecautiousofsecurityrisksbylimitingprivilegesandaccesstospecificIPs,ensuringstrongpasswords,andmonitori
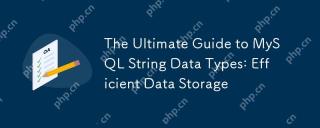
TostorestringsefficientlyinMySQL,choosetherightdatatypebasedonyourneeds:1)UseCHARforfixed-lengthstringslikecountrycodes.2)UseVARCHARforvariable-lengthstringslikenames.3)UseTEXTforlong-formtextcontent.4)UseBLOBforbinarydatalikeimages.Considerstorageov
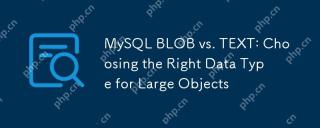
When selecting MySQL's BLOB and TEXT data types, BLOB is suitable for storing binary data, and TEXT is suitable for storing text data. 1) BLOB is suitable for binary data such as pictures and audio, 2) TEXT is suitable for text data such as articles and comments. When choosing, data properties and performance optimization must be considered.
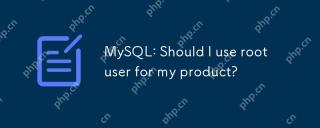
No,youshouldnotusetherootuserinMySQLforyourproduct.Instead,createspecificuserswithlimitedprivilegestoenhancesecurityandperformance:1)Createanewuserwithastrongpassword,2)Grantonlynecessarypermissionstothisuser,3)Regularlyreviewandupdateuserpermissions
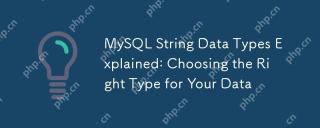
MySQLstringdatatypesshouldbechosenbasedondatacharacteristicsandusecases:1)UseCHARforfixed-lengthstringslikecountrycodes.2)UseVARCHARforvariable-lengthstringslikenames.3)UseBINARYorVARBINARYforbinarydatalikecryptographickeys.4)UseBLOBorTEXTforlargeuns


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
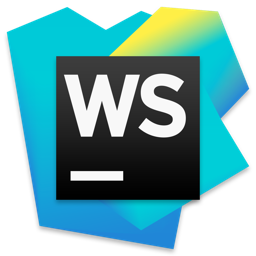
WebStorm Mac version
Useful JavaScript development tools
