


How to Unmarshal JSON Data into a Custom Struct with Specialized Substructures in Go?
Unmarshal JSON Data into a Custom Struct
In Go, unmarshaling JSON data into a struct is a common task. Consider the following JSON data:
<code class="json">{ "Asks": [[21, 1], [22, 1]], "Bids": [[20, 1], [19, 1]] }</code>
We can define a struct to represent this data:
<code class="go">type Message struct { Asks [][]float64 `json:"Asks"` Bids [][]float64 `json:"Bids"` }</code>
However, what if we want to further specialize the data structure, representing each order as an individual struct:
<code class="go">type Message struct { Asks []Order `json:"Asks"` Bids []Order `json:"Bids"` } type Order struct { Price float64 Volume float64 }</code>
Custom Unmarshaling with MarshalJSON
To achieve this, we can implement the json.Unmarshaler interface on our Order struct:
<code class="go">type Order struct { Price float64 Volume float64 } func (o *Order) UnmarshalJSON(data []byte) error { var v [2]float64 if err := json.Unmarshal(data, &v); err != nil { return err } o.Price = v[0] o.Volume = v[1] return nil }</code>
This method instructs Go to decode each element in the JSON as a 2-element array and assigns the values to the Price and Volume fields of the Order struct.
After implementing this method, we can now unmarshal the JSON data into our custom struct:
<code class="go">jsonBlob := []byte(`{"Asks": [[21, 1], [22, 1]], "Bids": [[20, 1], [19, 1]]}`) message := &Message{} if err := json.Unmarshal(jsonBlob, message); err != nil { panic(err) }</code>
Now, we can access the data using the custom Order struct:
<code class="go">fmt.Println(message.Asks[0].Price)</code>
The above is the detailed content of How to Unmarshal JSON Data into a Custom Struct with Specialized Substructures in Go?. For more information, please follow other related articles on the PHP Chinese website!
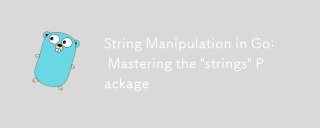
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
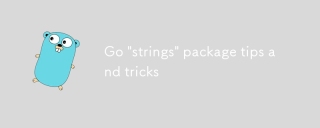
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
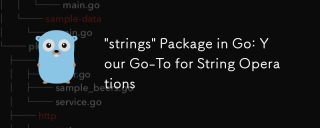
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
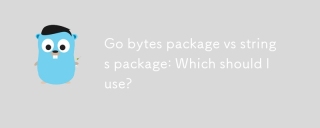
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
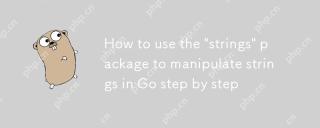
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
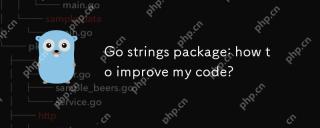
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
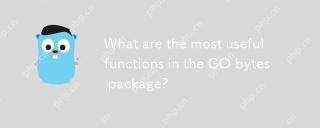
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
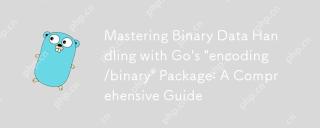
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
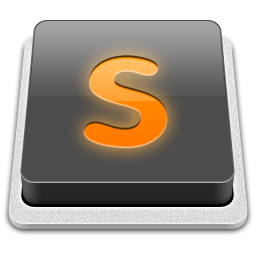
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
