Functions Implementing Interfaces in Go
In Go, interfaces define a set of methods that a type must implement to be considered a member of that interface. This allows for polymorphic behavior, as types implementing the same interface can be used interchangeably in code.
Example 1: Custom Type Implementing Interface
Consider the following interface for an HTTP handler:
type Handler interface { ServeHTTP(*Conn, *Request) }
The following type, Counter, implements this interface by providing a custom implementation of ServeHTTP:
type Counter int func (ctr *Counter) ServeHTTP(c *Conn, req *Request) { fmt.Fprintf(c, "counter = %d\n", ctr) ctr++ }
Example 2: Converting Function to Handler
The following function, notFound, does not implement the Handler interface directly:
func notFound(c *Conn, req *Request) { c.SetHeader("Content-Type", "text/plain;", "charset=utf-8") c.WriteHeader(StatusNotFound) c.WriteString("404 page not found\n") }
To make this function compatible with the Handler interface, Go provides a way to convert a function to a type with a specific method. In this case, the HandlerFunc type is defined:
type HandlerFunc func(*Conn, *Request)
A method, ServeHTTP, is added to this type:
func (f HandlerFunc) ServeHTTP(c *Conn, req *Request) { f(c, req) }
The notFound function can now be converted to an instance of HandlerFunc and assigned to a variable:
var Handle404 = HandlerFunc(notFound)
This allows Handle404 to be used as a Handler, even though the notFound function itself does not directly implement the Handler interface.
Explanation
In summary, Go allows functions to be converted to types with specific methods, addressing situations where a function does not directly fulfill an interface's requirements. By creating a type instance out of the function and defining a method on that type, the function can be accommodated by the interface, enabling polymorphic behavior in the program.
The above is the detailed content of How can functions be used to implement interfaces in Go?. For more information, please follow other related articles on the PHP Chinese website!
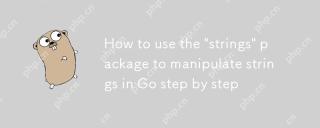
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
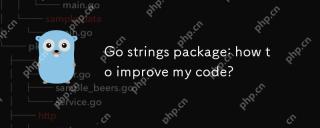
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
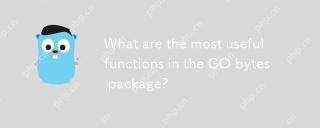
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
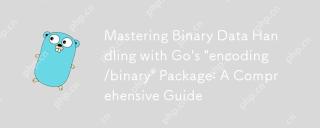
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
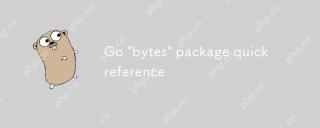
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
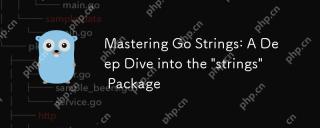
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
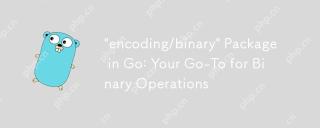
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
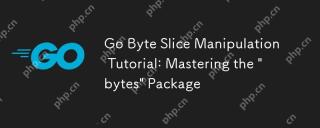
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
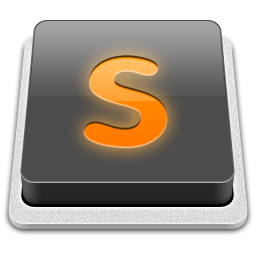
SublimeText3 Mac version
God-level code editing software (SublimeText3)
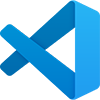
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
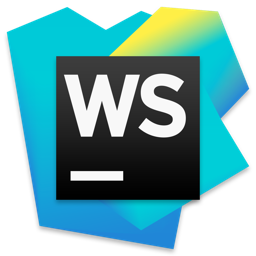
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
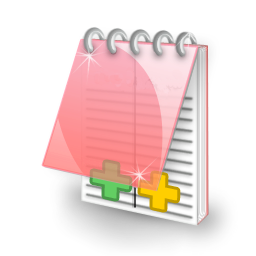
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
