Understanding Reference Parameters in C
Passing arguments by reference is a crucial concept in C programming. Referencing allows functions to modify the original variables passed to them, rather than operating on copies of those variables.
Why Use References?
Passing by reference is advantageous when:
- You want to modify the original variables that were passed to the function.
- You have large data structures that are expensive to copy. Passing by reference avoids duplicating these structures, improving performance.
How References Work
A reference is an alias to another variable. When you assign a reference to a variable, any operations performed on the reference directly affect the referenced variable. This is unlike passing by value, where a copy of the variable is created and any changes made to this copy do not affect the original variable.
Example
Consider the following functions:
int doSomething(int& a, int& b); int doSomething(int a, int b);
In the first function, a and b are references to the original variables passed to the function. Any changes made to a or b within the function will be reflected in the original variables.
However, in the second function, a and b are copies of the original variables. Any changes made to these copies will not affect the original variables.
Implications of Not Using References
If you do not make a parameter a reference, but instead leave off the &, the function will operate on a copy of the variable. In the context of the doSomething function above, this would mean that the following code:
int x = 2; int y = 3; doSomething(x, y);
Would not modify the original x and y variables. Instead, it would operate on copies of these variables within the function.
Conclusion
References are a powerful tool that allow functions to modify the original variables passed to them. This is particularly useful when working with large data structures or when it is necessary to modify the original variables. Understanding how to use references correctly is essential for effective C programming.
The above is the detailed content of When Should You Use References in C ?. For more information, please follow other related articles on the PHP Chinese website!

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
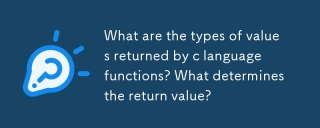
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing
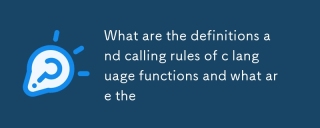
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
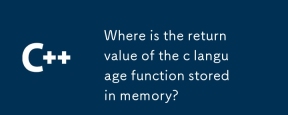
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
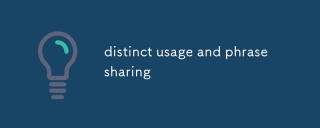
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
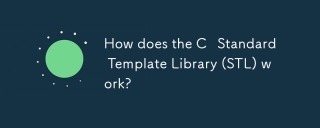
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
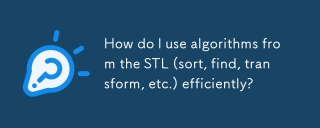
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
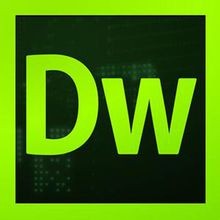
Dreamweaver CS6
Visual web development tools
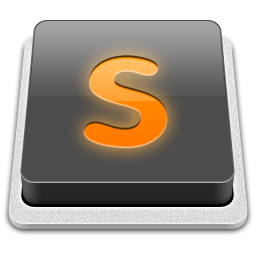
SublimeText3 Mac version
God-level code editing software (SublimeText3)
