Logging HTTP Request and Response Data
When developing web APIs in Go, logging both the incoming HTTP request and the outgoing HTTP response is crucial for monitoring and debugging. However, the default http.ResponseWriter interface does not provide a convenient way to capture the response data after it has been written.
Capturing Response Data Using io.MultiWriter
One solution is to use the io.MultiWriter function to create a writer that duplicates its writes to multiple destinations. This allows you to log the response while still sending it to the client.
<code class="go">func api1(w http.ResponseWriter, req *http.Request) { var log bytes.Buffer rsp := io.MultiWriter(w, &log) // Subsequent writes to rsp will be duplicated to both w and log ... }</code>
Capturing Request Data Using io.TeeReader
To capture the incoming HTTP request body for logging, you can use the io.TeeReader function to create a reader that reads from the original request body while also writing to a separate buffer.
<code class="go">func api1(w http.ResponseWriter, req *http.Request) { var log bytes.Buffer tee := io.TeeReader(req.Body, &log) err := json.NewDecoder(tee).Decode(&requestData) ... }</code>
Combining Request and Response Data for Logging
With both the request and response data captured in their respective buffers, you can now combine them into a single log message.
<code class="go">// Assuming we have set up log to be a logger with desired format and output log.Printf("%s %s %d %s %s", req.Method, req.URL.Path, req.Proto, log.BufioReader, log.Bytes())</code>
By combining these techniques, you can effectively capture and log both the incoming HTTP request and outgoing HTTP response data, providing valuable insights for troubleshooting and monitoring your API.
The above is the detailed content of How to Effectively Log HTTP Request and Response Data in Go APIs?. For more information, please follow other related articles on the PHP Chinese website!
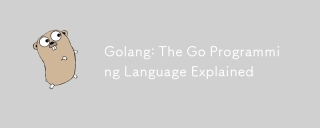
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
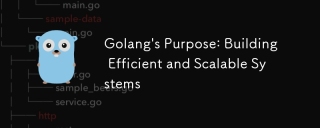
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
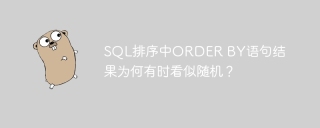
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
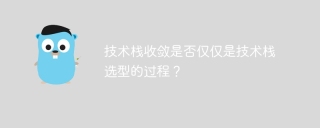
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
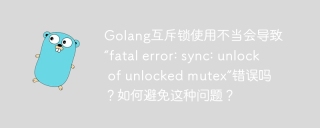
Golang ...
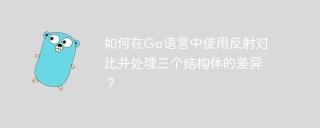
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...
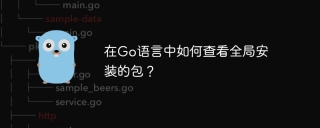
How to view globally installed packages in Go? In the process of developing with Go language, go often uses...
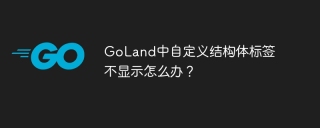
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
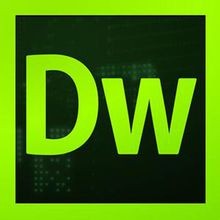
Dreamweaver CS6
Visual web development tools
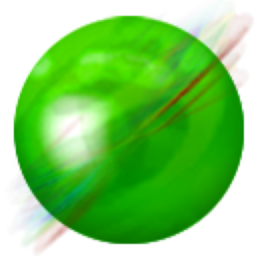
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
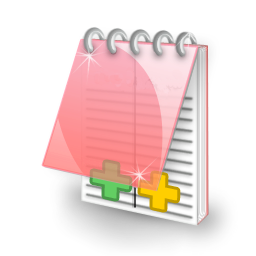
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function