Hello, community! Today, I want to introduce you to Lithe Mail, a package that simplifies SMTP email sending in PHP applications. It offers flexible integration with environment variables for easy configuration. Let’s take a look at how to set it up and use it in your project.
Installation
You can install the package via Composer. Run the following command in your terminal:
composer require lithemod/mail
Usage
Here’s a comprehensive guide on how to use the package to send emails:
1. Setting Up Environment Variables
Create a .env file in the root of your project and define your email settings:
MAIL_HOST=smtp.yourprovider.com MAIL_PORT=587 MAIL_USERNAME=your-email@domain.com MAIL_PASSWORD=your-password MAIL_ENCRYPTION=tls MAIL_FROM_ADDRESS=noreply@domain.com MAIL_FROM_NAME=Your Name or Company
2. Sending a Simple Text Email
<?php require 'vendor/autoload.php'; use Lithe\Support\Mail; use Lithe\Support\Env; // Load environment variables Env::load(__DIR__); // Send the email $mail = Mail::to('recipient@domain.com', 'Recipient Name') ->subject('Email Subject') ->text('Body of the email in plain text') ->send(); if ($mail) { echo 'Email sent successfully!'; } else { echo 'Failed to send email.'; }
3. Sending an HTML Email
<?php $mail = Mail::to('recipient@domain.com', 'Recipient Name') ->subject('Email Subject') ->html('<h1 id="Email-body-in-HTML">Email body in HTML</h1>') ->send(); if ($mail) { echo 'Email sent successfully!'; } else { echo 'Failed to send email.'; }
4. Adding CC and BCC Recipients
You can add CC and BCC recipients to your emails using the following methods:
Adding CC
$mail = Mail::to('recipient@domain.com', 'Recipient Name') ->cc('cc@example.com', 'CC Name') ->subject('Email Subject') ->text('Body of the email in plain text') ->send();
Adding BCC
$mail = Mail::to('recipient@domain.com', 'Recipient Name') ->bcc('bcc@example.com', 'BCC Name') ->subject('Email Subject') ->text('Body of the email in plain text') ->send();
5. Setting Reply-To Address
You can set a reply-to address using the replyTo method:
$mail = Mail::to('recipient@domain.com', 'Recipient Name') ->replyTo('replyto@example.com', 'Reply-To Name') ->subject('Email Subject') ->text('Body of the email in plain text') ->send();
6. Attaching Files
To attach files to your email, use the attach method:
$mail = Mail::to('recipient@domain.com', 'Recipient Name') ->subject('Email Subject') ->text('Body of the email in plain text') ->attach('/path/to/file.txt', 'CustomFilename.txt') ->send();
7. Adding Custom Headers
You can add custom headers to your email as follows:
$mail = Mail::to('recipient@domain.com', 'Recipient Name') ->subject('Email Subject') ->text('Body of the email in plain text') ->addHeader('X-Custom-Header', 'HeaderValue') ->send();
Final Thoughts
Lithe Mail offers a practical and efficient way to send emails in your PHP applications. With support for environment variables and various features, it can adapt to your email sending needs. Try it out and see how it can enhance communication in your application!
If you have any questions or suggestions, feel free to comment below!
The above is the detailed content of Lithe Mail: Simplifying Email Sending in PHP Applications. For more information, please follow other related articles on the PHP Chinese website!
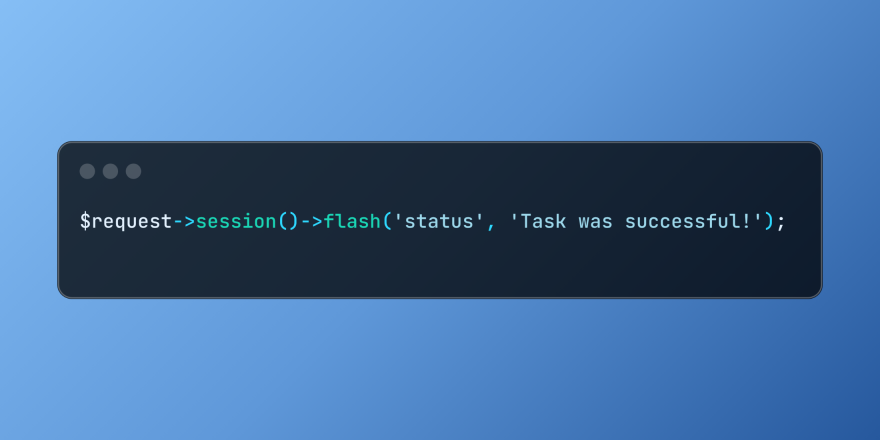
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
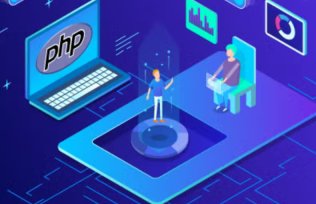
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
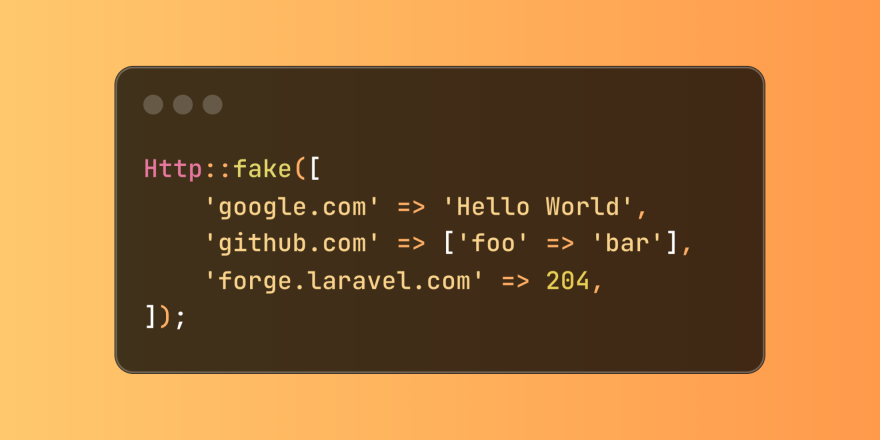
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
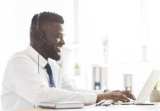
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
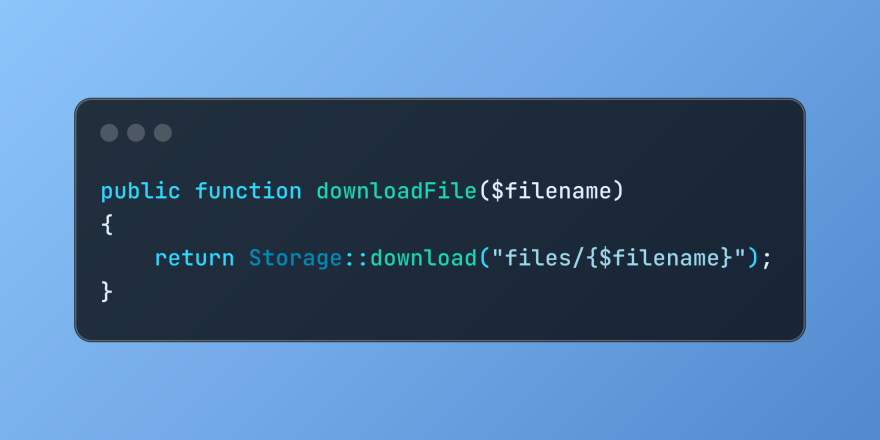
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
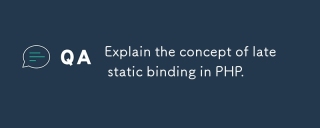
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
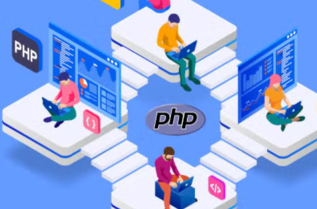
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
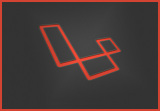
Laravel's service container and service providers are fundamental to its architecture. This article explores service containers, details service provider creation, registration, and demonstrates practical usage with examples. We'll begin with an ove


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
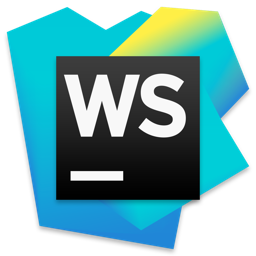
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
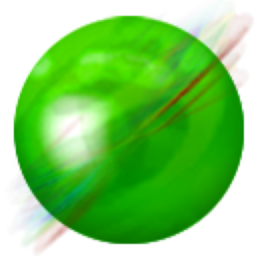
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
