


Connect to a Remote Server Using SSH with Private Key in Golang
Utilizing the SSH package in Go allows for convenient connections to remote servers through SSH protocols. However, establishing connections with private keys requires a deeper understanding of the package's functionality.
The SSH package provides support for various authentication methods, including password authentication. The Dial function, as mentioned in the package documentation, allows users to specify the authentication method to be employed in establishing the connection. To leverage a private key for authentication, we need to use additional methods provided by the package.
The PublicKeys function plays a crucial role in this scenario. It assists in converting a collection of SSH signers into an authentication mechanism. These signers can be obtained using various methods, including parsing them from PEM byte representations. The ability to utilize RSA, DSA, or ECDSA private keys directly is also supported through the use of the NewSignerFromKey function.
Let's consider an example that demonstrates the practical implementation of these concepts. This example incorporates support for agent-based authentication, which typically follows the utilization of private key authentication.
<code class="go">import ( "log" "net" "os" "golang.org/x/crypto/ssh" "golang.org/x/crypto/ssh/agent" ) func main() { // Establishes a connection to the SSH Agent sock, err := net.Dial("unix", os.Getenv("SSH_AUTH_SOCK")) if err != nil { log.Fatal(err) } agent := agent.NewClient(sock) // Acquires signers from the SSH Agent signers, err := agent.Signers() if err != nil { log.Fatal(err) } // Creates a list of authentication methods using the signers obtained auths := []ssh.AuthMethod{ssh.PublicKeys(signers...)} // Configures the client using the username and authentication details cfg := &ssh.ClientConfig{ User: "username", Auth: auths, } cfg.SetDefaults() // Establishes a connection to the remote server client, err := ssh.Dial("tcp", "aws-hostname:22", cfg) if err != nil { log.Fatal(err) } // Creates a new session in the established connection session, err = client.NewSession() if err != nil { log.Fatal(err) } log.Println("Successfully established a session!") }</code>
With this enhanced code sample, it's possible to connect to a server remotely using a private key through the SSH package in Go. Additional features like agent-based authentication have also been incorporated for a more comprehensive demonstration of the entire process.
The above is the detailed content of How to Connect to a Remote Server Using SSH with a Private Key in Golang?. For more information, please follow other related articles on the PHP Chinese website!
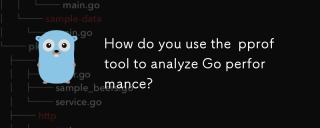
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
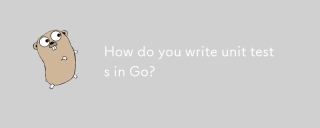
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
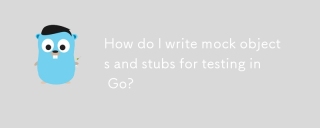
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
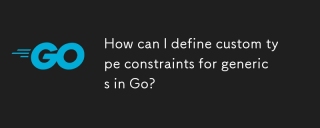
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
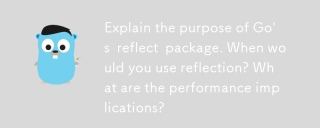
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
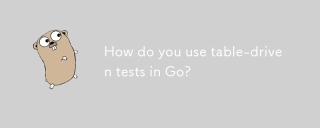
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
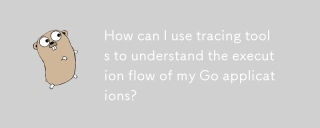
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
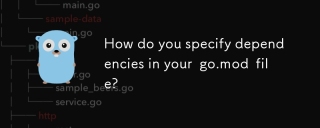
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
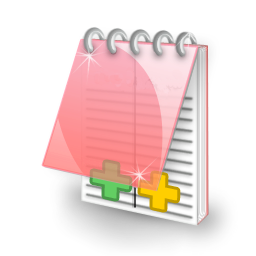
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
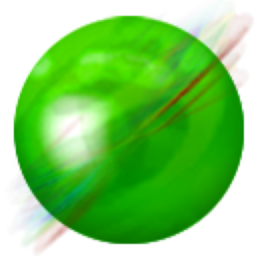
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
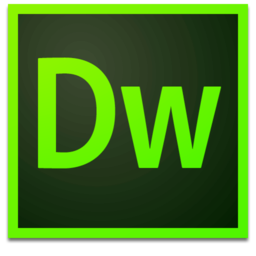
Dreamweaver Mac version
Visual web development tools
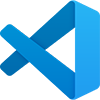
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
