Implicit Conversion of Strongly Typed Enums to Int
Strongly typed enums, introduced in C 11, are designed to prevent implicit conversions to integers. However, there may be cases where you want to convert a strongly typed enum value to an int without an explicit cast.
To address this, several approaches have been suggested:
- Using a Function:
You can define a function that performs the conversion. For instance:
<code class="cpp">#include <iostream> struct a { enum LOCAL_A { A1, A2 }; }; template <typename e> int to_int(E e) { return static_cast<int>(e); } int main() { // Use the to_int function to convert the strongly typed enum value b::B2 to int std::cout <ol start="2"><li><strong>Using a Function with Template Argument Deduction:</strong></li></ol> <p>To simplify the syntax, you can use a function template that automatically deduces the enum type:</p> <pre class="brush:php;toolbar:false"><code class="cpp">#include <iostream> struct a { enum LOCAL_A { A1, A2 }; }; template <typename e> constexpr typename std::underlying_type<e>::type get_underlying(E e) noexcept { return static_cast<typename std::underlying_type>::type>(e); } int main() { // Use the get_underlying function to convert the strongly typed enum value b::B2 to int std::cout <ol start="3"><li><strong>Creating a Macro:</strong></li></ol> <p>You can also utilize a macro to make the conversion process more concise:</p> <pre class="brush:php;toolbar:false"><code class="cpp">#include <iostream> struct a { enum LOCAL_A { A1, A2 }; }; #define TO_INT(e) static_cast<int>(e) int main() { // Use the TO_INT macro to convert the strongly typed enum value b::B2 to int std::cout <p>While it's possible to convert strongly typed enums to ints without explicit casts, it's important to note that this may lead to unintended behavior. It's recommended to use explicit casts whenever appropriate to avoid potential issues.</p></int></iostream></code>
The above is the detailed content of How to Implicitly Convert Strongly Typed Enums to Ints in C ?. For more information, please follow other related articles on the PHP Chinese website!
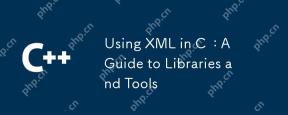
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
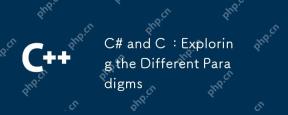
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
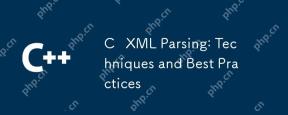
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
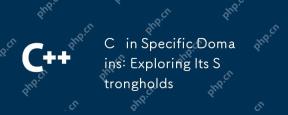
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
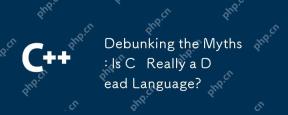
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
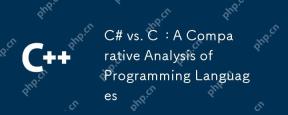
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
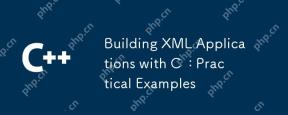
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
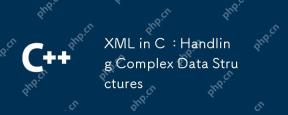
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
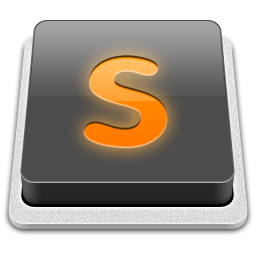
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment
