


Separating Text Without Spaces into a Word List
Overview
Given a string consisting of words without spaces, this article presents an efficient algorithm for segmenting it into individual words while considering their relative frequencies.
Problem Statement
Input: "tableapplechairtablecupboard..."
Output: ["table", "apple", "chair", "table", ["cupboard", ["cup", "board"]], ...]
Algorithm Overview
Rather than using a naive approach, the algorithm leverages word frequency to improve accuracy. Assuming words are distributed independently and follow Zipf's law, the algorithm uses dynamic programming to identify the most likely sequence of words.
The Code
<code class="python">from math import log words = open("words-by-frequency.txt").read().split() wordcost = dict((k, log((i+1)*log(len(words)))) for i,k in enumerate(words)) maxword = max(len(x) for x in words) def infer_spaces(s): cost = [0] for i in range(1,len(s)+1): c,k = best_match(i) cost.append(c) out = [] i = len(s) while i>0: c,k = best_match(i) out.append(s[i-k:i]) i -= k return " ".join(reversed(out)) def best_match(i): candidates = enumerate(reversed(cost[max(0, i-maxword):i])) return min((c + wordcost.get(s[i-k-1:i], 9e999), k+1) for k,c in candidates) s = 'thumbgreenappleactiveassignmentweeklymetaphor' print(infer_spaces(s))</code>
Word Frequency Estimation
The algorithm relies on a dictionary that maps words to their relative frequencies, assuming Zipf's law. To account for unseen words, a high cost is assigned to them.
Dynamic Programming
The algorithm computes the cost of each possible word segment, considering the potential next words. It selects the path with the lowest cost using dynamic programming, ensuring the most likely word sequence.
Performance Optimization
For large inputs, the algorithm can be optimized by splitting the text into blocks and processing them independently. This reduces memory usage without significantly impacting accuracy.
The above is the detailed content of How can we efficiently separate text without spaces into a word list, leveraging word frequency and dynamic programming?. For more information, please follow other related articles on the PHP Chinese website!
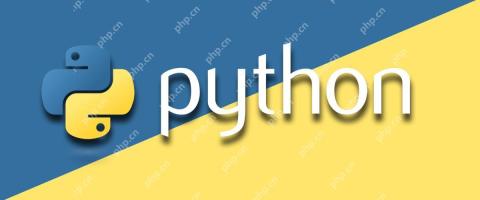
Implementing factory pattern in Python can create different types of objects by creating a unified interface. The specific steps are as follows: 1. Define a basic class and multiple inheritance classes, such as Vehicle, Car, Plane and Train. 2. Create a factory class VehicleFactory and use the create_vehicle method to return the corresponding object instance according to the type parameter. 3. Instantiate the object through the factory class, such as my_car=factory.create_vehicle("car","Tesla"). This pattern improves the scalability and maintainability of the code, but it needs to be paid attention to its complexity
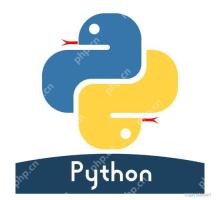
In Python, the r or R prefix is used to define the original string, ignoring all escaped characters, and letting the string be interpreted literally. 1) Applicable to deal with regular expressions and file paths to avoid misunderstandings of escape characters. 2) Not applicable to cases where escaped characters need to be preserved, such as line breaks. Careful checking is required when using it to prevent unexpected output.
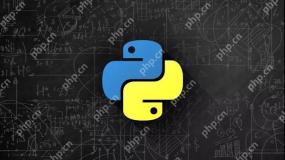
In Python, the __del__ method is an object's destructor, used to clean up resources. 1) Uncertain execution time: Relying on the garbage collection mechanism. 2) Circular reference: It may cause the call to be unable to be promptly and handled using the weakref module. 3) Exception handling: Exception thrown in __del__ may be ignored and captured using the try-except block. 4) Best practices for resource management: It is recommended to use with statements and context managers to manage resources.
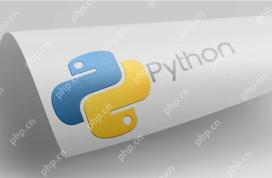
The pop() function is used in Python to remove elements from a list and return a specified position. 1) When the index is not specified, pop() removes and returns the last element of the list by default. 2) When specifying an index, pop() removes and returns the element at the index position. 3) Pay attention to index errors, performance issues, alternative methods and list variability when using it.
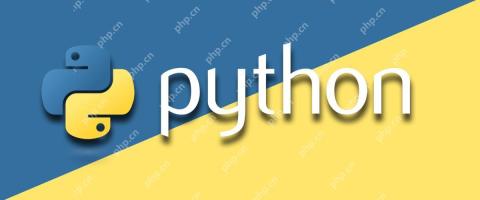
Python mainly uses two major libraries Pillow and OpenCV for image processing. Pillow is suitable for simple image processing, such as adding watermarks, and the code is simple and easy to use; OpenCV is suitable for complex image processing and computer vision, such as edge detection, with superior performance but attention to memory management is required.
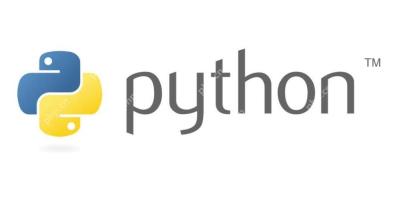
Implementing PCA in Python can be done by writing code manually or using the scikit-learn library. Manually implementing PCA includes the following steps: 1) centralize the data, 2) calculate the covariance matrix, 3) calculate the eigenvalues and eigenvectors, 4) sort and select principal components, and 5) project the data to the new space. Manual implementation helps to understand the algorithm in depth, but scikit-learn provides more convenient features.
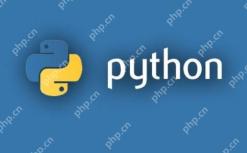
Calculating logarithms in Python is a very simple but interesting thing. Let's start with the most basic question: How to calculate logarithm in Python? Basic method of calculating logarithm in Python The math module of Python provides functions for calculating logarithm. Let's take a simple example: importmath# calculates the natural logarithm (base is e) x=10natural_log=math.log(x)print(f"natural log({x})={natural_log}")# calculates the logarithm with base 10 log_base_10=math.log10(x)pri
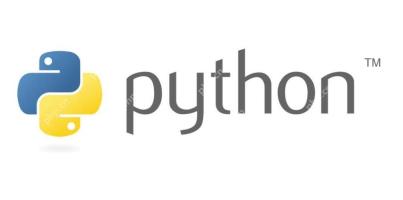
To implement linear regression in Python, we can start from multiple perspectives. This is not just a simple function call, but involves a comprehensive application of statistics, mathematical optimization and machine learning. Let's dive into this process in depth. The most common way to implement linear regression in Python is to use the scikit-learn library, which provides easy and efficient tools. However, if we want to have a deeper understanding of the principles and implementation details of linear regression, we can also write our own linear regression algorithm from scratch. The linear regression implementation of scikit-learn uses scikit-learn to encapsulate the implementation of linear regression, allowing us to easily model and predict. Here is a use sc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
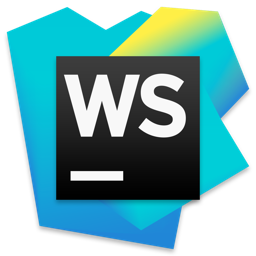
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
