Image to ASCII art conversion in C
Converting a bitmap image to ASCII art involves using mono-spaced fonts and keeping the process simple. There are two main approaches:
Pixel/area intensity based:
This approach treats each pixel or area of pixels as a single dot. It computes the average gray scale intensity of the dot and replaces it with a character of closest intensity.
Character fitting (hybrid):
This approach tries to replace areas (character shaped) with characters of similar intensity and shape. It leads to better results but is slower due to computations.
Detailed Implementation:
Pixel/area intensity based:
Divide the image into gray-scale pixels or rectangular areas (dots). Compute the intensity of each dot. Replace it with a character from a character map (precomputed intensities) with the closest intensity.
A sample character map: " .,:;ox%#@&"
Intensity computation:
<code class="C++">i = p[x+x+x+0]; i += p[x+x+x+1]; i += p[x+x+x+2]; i = (i*l)/768; s += m[l-i];</code>
where:
- i is the summation of pixel intensities
- l is the length of the character map
- m is the character map
- s is the output string
Character fitting:
Divide the image into rectangular areas with the same aspect ratio as the characters. Divide the character area into zones and compute the intensity of each zone. Find the character in the map with the closest intensity and shape fit.
This approach leads to best, most visually pleasing results when using larger fonts.
Code Example:
<code class="C++">class intensity { public: char c; // Character int il, ir, iu ,id, ic; // Intensity of part: left,right,up,down,center intensity() { c=0; reset(); } void reset() { il=0; ir=0; iu=0; id=0; ic=0; } void compute(DWORD **p,int xs,int ys,int xx,int yy) // p source image, (xs,ys) area size, (xx,yy) area position { int x0 = xs>>2, y0 = ys>>2; int x1 = xs-x0, y1 = ys-y0; int x, y, i; reset(); for (y=0; y<ys y for x i="(p[yy+y][xx+x]" if il>=x1) ir+=i; if (y=x1) id+=i; if ((x>=x0) && (x=y0) && (yWidth; ys = bmp->Height; // Output font size xf = font->Size; if (xfHeight; if (yfHandleType = bmDIB; bmp->HandleType = bmDIB; tmp->PixelFormat = pf32bit; bmp->PixelFormat = pf32bit; // Copy target font properties to tmp tmp->Canvas->Font->Assign(font); tmp->SetSize(xf, yf); tmp->Canvas->Font ->Color = clBlack; tmp->Canvas->Pen ->Color = clWhite; tmp->Canvas->Brush->Color = clWhite; xf = tmp->Width; yf = tmp->Height; // Direct pixel access to bitmaps p = new DWORD*[ys]; if (p == NULL) break; for (y=0; y<ys y p>ScanLine[y]; q = new DWORD*[yf]; if (q == NULL) break; for (y=0; y<yf y q>ScanLine[y]; // Create character map for (x=0, d=32; dCanvas->FillRect(TRect(0, 0, xf, yf)); // Render tested character to tmp tmp->Canvas->TextOutA(0, 0, map[x].c); // Compute intensity map[x].compute(q, xf, yf, 0, 0); } map[x].c = 0; // Loop through the image by zoomed character size step xf -= xf/3; // Characters are usually overlapping by 1/3 xs -= xs % xf; ys -= ys % yf; for (y=0; y<ys y txt eol for x compute intensity gfx.compute xf yf find the closest match in map i0="0;" d0="-1;" i d="abs(map[i].il-gfx.il)" abs if>d)) { d0=d; i0=i; } } // Add fitted character to output txt += map[i0].c; } break; } // Free buffers if (tmp) delete tmp; if (p ) delete[] p; return txt; }</ys></yf></ys></ys></code>
The above is the detailed content of How do you convert a bitmap image to ASCII art in C ?. For more information, please follow other related articles on the PHP Chinese website!

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
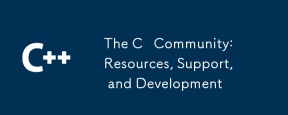
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
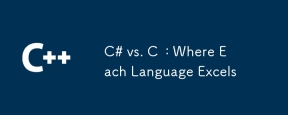
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
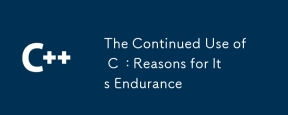
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
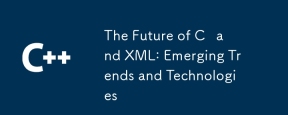
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
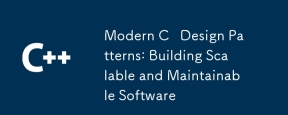
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
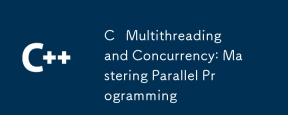
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
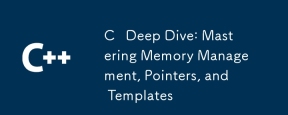
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),