


Session Variables in Golang Not Saved While Using Gorilla Sessions
Issue Description
In a Gorilla sessions implementation, session variables are not being maintained across requests. After logging in and setting the session variable, a new tab should maintain the session, but instead, users are redirected to the login page.
Code Analysis
<code class="go">sessionNew.Save(req, res)</code>
This code is missing error handling for sessionNew.Save(). If the saving process fails, the error will be ignored, leading to unexpected behavior. It should be updated to:
<code class="go">err := sessionNew.Save(req, res) if err != nil { // Handle the error }</code>
Session Path
The session path is set to "/loginSession", which limits the session's scope to only that specific path. This can cause confusion because users visiting other routes will not have access to the session. To ensure the session is available across all routes, the path should be set to "/".
Session Check
In SessionHandler, the session check is performed after serving static files. This can cause issues because the static files are served before the session is validated. The session check should be performed before serving any content.
Complete Code with Improvements
<code class="go">package main import ( "crypto/md5" "encoding/hex" "fmt" "github.com/gocql/gocql" "github.com/gorilla/mux" "github.com/gorilla/sessions" "net/http" "time" ) var store = sessions.NewCookieStore([]byte("something-very-secret")) var router = mux.NewRouter() func init() { store.Options = &sessions.Options{ Domain: "localhost", Path: "/", MaxAge: 3600 * 8, // 8 hours HttpOnly: true, } } func main() { //session handling router.HandleFunc("/", sessionHandler) router.HandleFunc("/signIn", signInHandler) router.HandleFunc("/signUp", signUpHandler) router.HandleFunc("/logOut", logOutHandler) http.Handle("/", router) http.ListenAndServe(":8100", nil) } //handler for signIn func signInHandler(res http.ResponseWriter, req *http.Request) { // Get the session session, err := store.Get(req, "loginSession") if err != nil { // Handle the error } // Set session values session.Values["email"] = req.FormValue("email") session.Values["name"] = req.FormValue("password") // Save the session err = session.Save(req, res) if err != nil { // Handle the error } } //handler for signUp func signUpHandler(res http.ResponseWriter, req *http.Request) { // ... } //handler for logOut func logOutHandler(res http.ResponseWriter, req *http.Request) { // Get the session session, err := store.Get(req, "loginSession") if err != nil { // Handle the error } // Save the session (with updated values) err = session.Save(req, res) if err != nil { // Handle the error } } //handler for Session func sessionHandler(res http.ResponseWriter, req *http.Request) { // Get the session session, err := store.Get(req, "loginSession") if err != nil { // Handle the error } // Check if the session is valid if session.Values["email"] == nil { http.Redirect(res, req, "html/login.html", http.StatusFound) } else { http.Redirect(res, req, "html/home.html", http.StatusFound) } }</code>
The above is the detailed content of Why Are My Golang Session Variables Not Being Saved in Gorilla Sessions?. For more information, please follow other related articles on the PHP Chinese website!
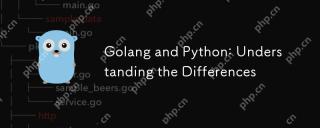
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
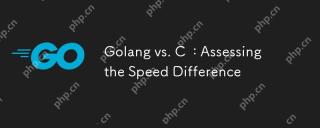
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
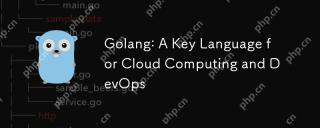
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
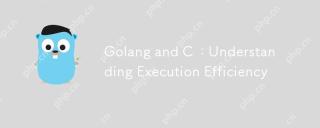
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
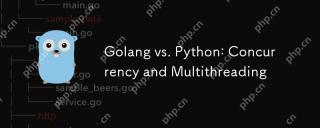
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
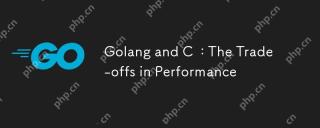
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
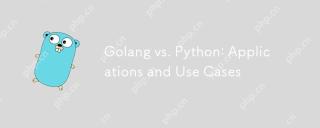
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
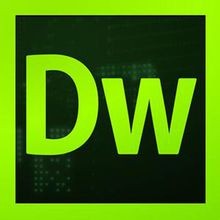
Dreamweaver CS6
Visual web development tools