Determining the Number of Rows Returned by a MySQL Query
Counting the number of rows returned by a MySQL query is essential for pagination, data analysis, and other scenarios. Here are various methods to achieve this:
Method 1: Using mysql_num_rows for Iterative Counting
For PHP applications, you can utilize the mysql_num_rows function to determine the number of rows in a result set. This function is exposed through the mysqli_num_rows function in PHP, as exemplified below:
<code class="php">$link = mysqli_connect("localhost", "user", "password", "database"); $result = mysqli_query($link, "SELECT * FROM table1"); $num_rows = mysqli_num_rows($result); echo "$num_rows Rows\n";</code>
Method 2: COUNT(*) Function for Matching Criteria
To count the number of rows that meet specific criteria, use the COUNT(*) function in your query. For instance:
<code class="sql">SELECT COUNT(*) FROM foo WHERE bar='value';</code>
Method 3: SQL_CALC_FOUND_ROWS for Total Rows with LIMIT
If you're using a LIMIT clause and need the total number of rows without the limit, you can employ the SQL_CALC_FOUND_ROWS and FOUND_ROWS() functions:
<code class="sql">SELECT SQL_CALC_FOUND_ROWS * FROM foo WHERE bar="value" LIMIT 10; SELECT FOUND_ROWS();</code>
Note: SQL_CALC_FOUND_ROWS is deprecated as of MySQL 8.0.17 and is recommended to be replaced with a separate query to obtain a count.
The above is the detailed content of How to Count the Number of Rows Returned by a MySQL Query?. For more information, please follow other related articles on the PHP Chinese website!
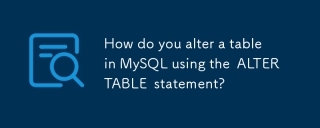
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
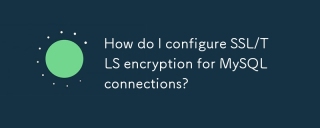
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
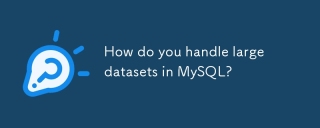
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
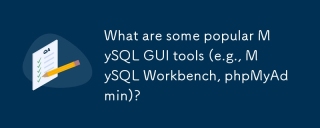
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
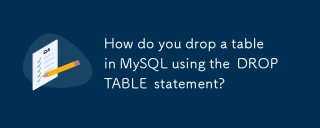
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
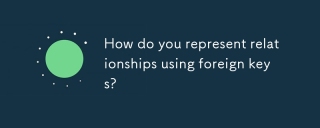
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
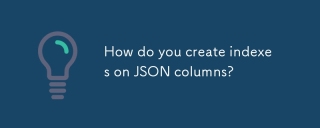
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
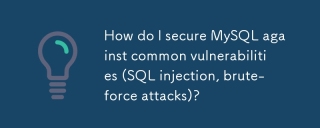
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
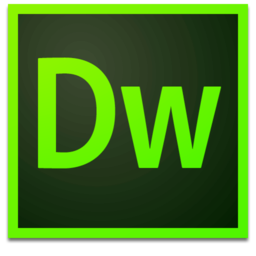
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
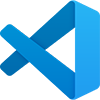
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
