Infinity Loop Slider Concepts
Developing an infinite loop slider for a website using JavaScript/jQuery requires careful architectural considerations to ensure code efficiency, readability, best practices, and reusability. Understanding the best approach to arrange images for the impression of an infinite loop is crucial.
Image Arrangement for Infinity Loop
Examining different slider implementations has revealed two primary solutions:
- Changing Z-Index of Images: This method dynamically alters the Z-index of each image as the next/previous image is displayed.
- Changing DOM Position of Images: This approach manipulates the position of images within the DOM to create the infinite loop effect.
Optimal Architectural Approach
A simple and effective approach involves cloning the first and last images to create a seamless infinite loop:
- Clone Last Image Before First: Prepend the clone of the last image before the first image in the DOM.
- Clone First Image After Last: Append the clone of the first image after the last image in the DOM.
This arrangement ensures that when the user switches from the last image to the first or vice versa, the cloned image acts as a placeholder, creating the illusion of an infinite loop.
JavaScript/jQuery Implementation
The following JavaScript/jQuery code snippet demonstrates how to implement this approach:
<code class="javascript">// Clone the first and last images first.before(last.clone(true)); last.after(first.clone(true)); // Handle navigation buttons triggers.on('click', function(e) { var delta = (e.target.id === 'prev')? -1 : 1; gallery.animate({ left: `+=${-100 * delta}` }, function() { current += delta; // Handle cycling if (current === 0 || current > len) { current = (current === 0)? len : 1; gallery.css({ left: -100 * current }); } }); });</code>
Conclusion
This approach provides an efficient and straightforward solution for creating an infinity loop slider. It involves minimal DOM manipulation and JavaScript logic, ensuring code readability, best practices, and reusability.
The above is the detailed content of How to Achieve an Infinite Loop Slider Effect with JavaScript/jQuery?. For more information, please follow other related articles on the PHP Chinese website!
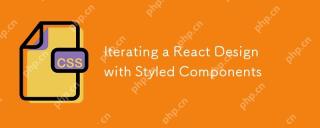
In a perfect world, our projects would have unlimited resources and time. Our teams would begin coding with well thought out and highly refined UX designs.
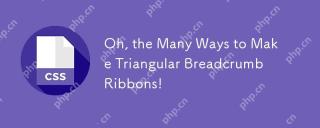
Oh, the Many Ways to Make Triangular Breadcrumb Ribbons
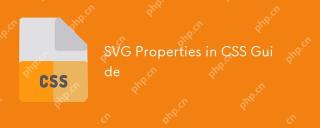
SVG has its own set of elements, attributes and properties to the extent that inline SVG code can get long and complex. By leveraging CSS and some of the forthcoming features of the SVG 2 specification, we can reduce that code for cleaner markup.
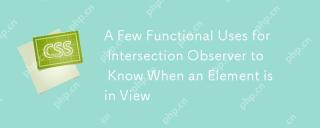
You might not know this, but JavaScript has stealthily accumulated quite a number of observers in recent times, and Intersection Observer is a part of that
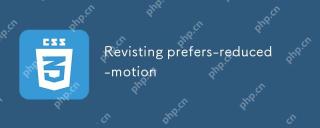
We may not need to throw out all CSS animations. Remember, it’s prefers-reduced-motion, not prefers-no-motion.
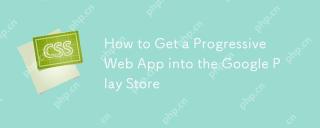
PWA (Progressive Web Apps) have been with us for some time now. Yet, each time I try explaining it to clients, the same question pops up: "Will my users be
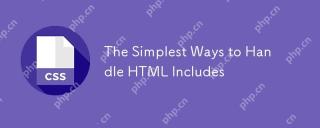
It's extremely surprising to me that HTML has never had any way to include other HTML files within it. Nor does there seem to be anything on the horizon that
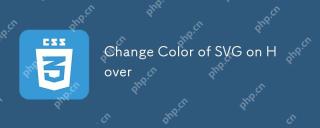
There are a lot of different ways to use SVG. Depending on which way, the tactic for recoloring that SVG in different states or conditions — :hover,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
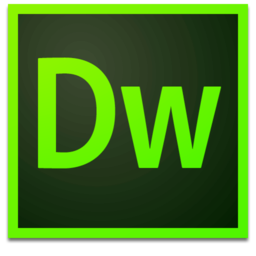
Dreamweaver Mac version
Visual web development tools
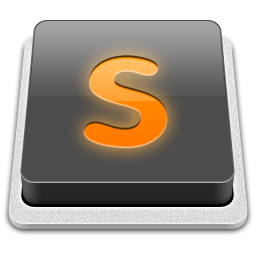
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
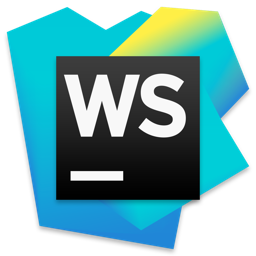
WebStorm Mac version
Useful JavaScript development tools