Singleton Pattern in C with Proper Memory Management
The Singleton pattern ensures that only one instance of a class can be created. While many approaches exist, a common practice is to use a static pointer to manage the instance creation and destruction. However, it's crucial to adhere to memory management rules to prevent errors.
In this example:
<code class="cpp">class A { static A* m_pA; A(); ~A(); static A* GetInstance(); static void FreeInstance(); void WORK1(); void WORK2(); void WORK3(); }; A* A::GetInstance() { if (m_pA == NULL) m_pA = new A(); return m_pA; } A::~A() { FreeInstance() // Don't write this here } void A::FreeInstance() { delete m_pA; m_pA = NULL; }</code>
Manually freeing a singleton using the destructor is incorrect. If external references to the instance exist, deleting it prematurely will lead to crashes.
Instead, adopt a better approach:
<code class="cpp">class A { static A* m_pA; explicit A(); void A(const A& a); void A(A &a); const A& operator=(const A& a); ~A(); static A* GetInstance(); static void FreeInstance(); void WORK1(); void WORK2(); void WORK3(); }; A* A::GetInstance() { if (m_pA == NULL){ static A self; m_pA = &self; } return m_pA; } A::~A() { }</code>
Here, the static instance is created and destroyed automatically by the compiler, ensuring proper memory management. Additionally, mark the constructor as explicit to prevent implicit type conversions and make the copy constructor and assignment operator private to prohibit undesired object duplication.
The above is the detailed content of How to Achieve Proper Memory Management in C Singleton Implementation?. For more information, please follow other related articles on the PHP Chinese website!
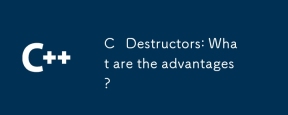
C destructorsprovideseveralkeyadvantages:1)Theymanageresourcesautomatically,preventingleaks;2)Theyenhanceexceptionsafetybyensuringresourcerelease;3)TheyenableRAIIforsaferesourcehandling;4)Virtualdestructorssupportpolymorphiccleanup;5)Theyimprovecode
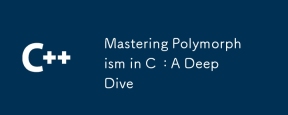
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
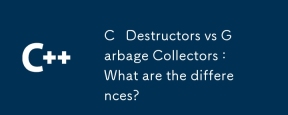
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
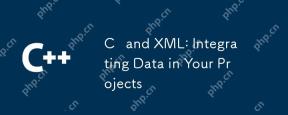
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
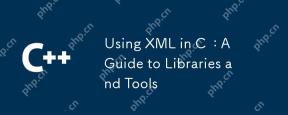
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
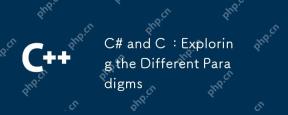
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
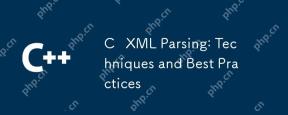
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
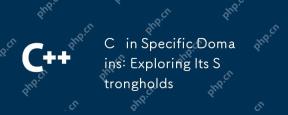
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
