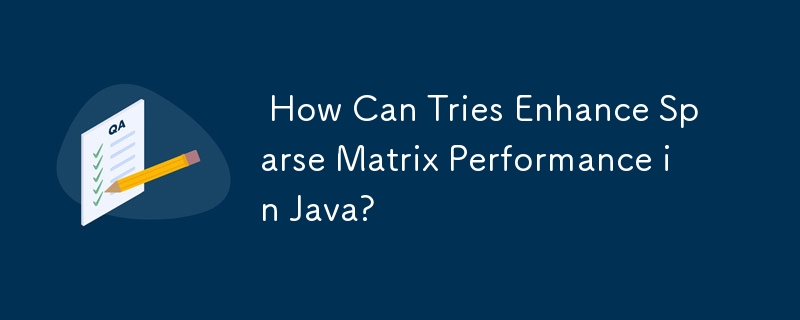
Sparse Matrices / Arrays in Java
Hashmaps built with sparse arrays are inefficient for data that is read frequently. The most efficient way to implement sparse arrays is to use a Trie, which allows for fast access to a single vector where segments are distributed.
Using a Trie
A Trie can determine if an element exists in the table with only two read-only array indexing operations, providing the effective position of the element or indicating its absence. It can also provide a default position in the backing store for the default value of the sparse array, eliminating the need for any test on the returned index.
Vorteile of Tries
- Much faster than hashmaps due to no complex hashing functions and no collision handling
- Java Hashmaps can only index objects, while Tries can handle integers
- Memory-efficient, as integer object creation for each hashed source index is not required
Steps for Implementing a Sparse Array with a Trie
- Define subrange and offset computations using subrangeOf() and positionOffsetOf() methods.
- Use system.arraycompare() and system.arraycopy() for efficient array operations.
- Set up the Trie structure and its internal members.
- Provide methods for reset, setting values (setAt()), and getting values (getAt()).
- Optionally implement a compact() method to optimize storage by detecting and merging common subranges.
Vorteile of Implementing Sparse Arrays with Tries
- Fast reallocation of new subranges
- Automatic detection and compression of subranges
- Shared data for common subranges
- Read-only data for shared subranges
Limitations
- The current implementation has static subrange sizes, limiting flexibility.
- Compaction is limited to detecting common subranges without interleaving.
Additional Considerations
- Colt library is good but not optimized for sparse matrices, using hashing techniques.
- Trove implementation is also based on hashing techniques with similar limitations.
- Tries offer superior speed and moderate space consumption compared to hashing and row-compressed techniques.
The above is the detailed content of How Can Tries Enhance Sparse Matrix Performance in Java?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn