How to Detect Long Clicks in Android Using OpenGL-ES
To detect when the user presses a surface being rendered by an OpenGL-ES application, developers typically use the onTouchEvent(MotionEvent event) method. However, this method does not have built-in functionality for detecting long clicks.
One approach is to register for the ACTION_DOWN event. Then, in onTouchEvent, schedule a Runnable to run after a certain time delay. If the Runnable is canceled before it runs, due to an ACTION_UP or ACTION_MOVE event, it indicates that the user did not perform a long click.
Alternatively, Android provides a more sophisticated solution: GestureDetector, which can be used to detect a variety of gestures, including long clicks.
Using GestureDetector
To use GestureDetector, follow these steps:
- Create an instance of GestureDetector.
- Override the onTouchEvent method in your activity or fragment.
- In onTouchEvent, pass the touch event to the GestureDetector instance using gestureDetector.onTouchEvent(event).
- Register a OnGestureListener with the GestureDetector to detect long clicks.
Here is an example of using GestureDetector to detect long clicks:
<code class="kotlin">class MyActivity : AppCompatActivity() { private lateinit var gestureDetector: GestureDetector override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) gestureDetector = GestureDetector(this, LongClickListener()) } override fun onTouchEvent(event: MotionEvent): Boolean { gestureDetector.onTouchEvent(event) return super.onTouchEvent(event) } inner class LongClickListener : OnGestureListener { override fun onLongPress(e: MotionEvent?) { // Handle long click here. } // Implement other gesture methods as needed. } }</code>
By using GestureDetector, you can easily detect long clicks in your OpenGL-ES applications.
The above is the detailed content of How to Detect Long Clicks in Android OpenGL-ES Applications?. For more information, please follow other related articles on the PHP Chinese website!
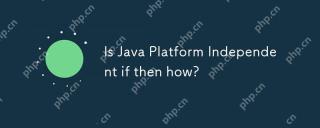
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
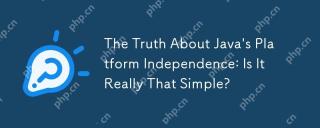
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
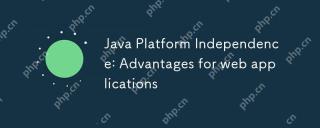
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
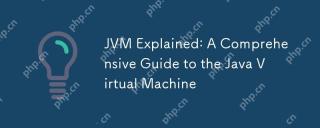
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
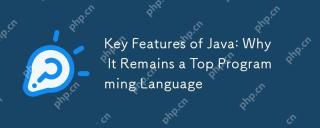
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
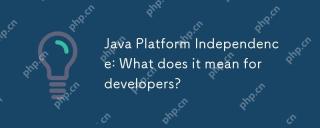
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
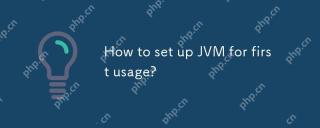
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
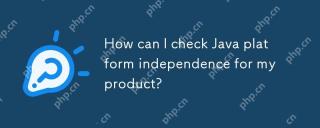
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
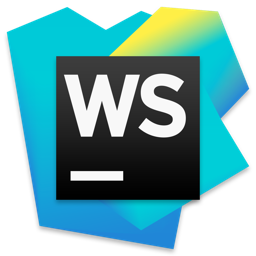
WebStorm Mac version
Useful JavaScript development tools
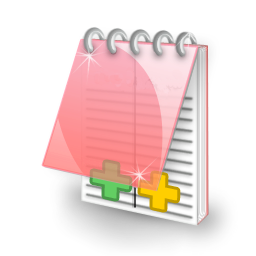
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
