


How can I merge JavaScript objects with common keys into a new array where values are combined?
Merging JavaScript Objects with Common Key
In JavaScript, merging objects can be straightforward. However, the process becomes more complex when dealing with an array of objects where multiple objects share a common key and their values need to be combined. Consider the example below:
<code class="js">var array = [ { name: "foo1", value: "val1" }, { name: "foo1", value: ["val2", "val3"] }, { name: "foo2", value: "val4" } ];</code>
The goal is to reorganize the array into a format where all values associated with the same name key are merged together. The expected output would look like this:
<code class="js">var output = [ { name: "foo1", value: ["val1", "val2", "val3"] }, { name: "foo2", value: ["val4"] } ];</code>
To achieve this merging, one effective approach involves iterating through the input array and filtering for existing objects with the same name key. If an existing object is found, its value is updated to include the new value. Otherwise, a new object is created and added to the output array.
Here's an example implementation:
<code class="js">var output = []; array.forEach(function(item) { var existing = output.filter(function(v, i) { return v.name == item.name; }); if (existing.length) { var existingIndex = output.indexOf(existing[0]); output[existingIndex].value = output[existingIndex].value.concat(item.value); } else { if (typeof item.value == 'string') item.value = [item.value]; output.push(item); } });</code>
This approach ensures that the values associated with each name key are correctly merged into a single object within the output array. By iterating through the input array and handling both existing and new objects, it provides a comprehensive solution for merging objects with common keys.
The above is the detailed content of How can I merge JavaScript objects with common keys into a new array where values are combined?. For more information, please follow other related articles on the PHP Chinese website!
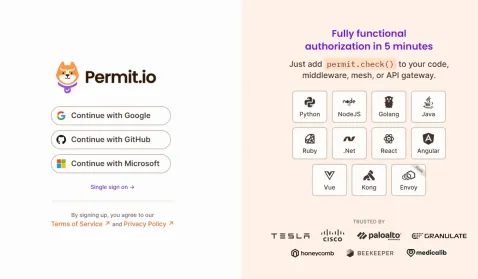
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
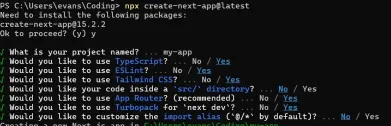
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
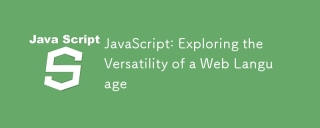
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
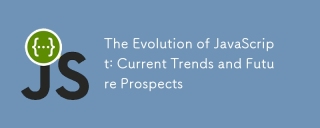
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
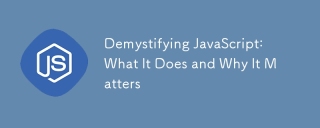
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
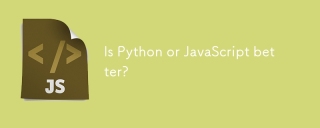
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.
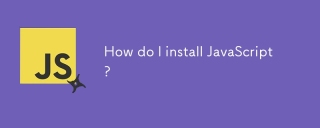
JavaScript does not require installation because it is already built into modern browsers. You just need a text editor and a browser to get started. 1) In the browser environment, run it by embedding the HTML file through tags. 2) In the Node.js environment, after downloading and installing Node.js, run the JavaScript file through the command line.
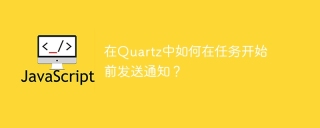
How to send task notifications in Quartz In advance When using the Quartz timer to schedule a task, the execution time of the task is set by the cron expression. Now...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
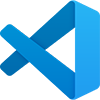
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),