Accessing Multiple gRPC Services over a Single Connection
gRPC is a popular remote procedure call (RPC) framework for building distributed systems. When accessing multiple gRPC services, it's tempting to establish a socket connection for each service. However, this approach can lead to inefficient resource utilization.
To address this, gRPC allows you to multiplex multiple services over a single connection. This不仅 can improve efficiency, but also simplifies your code.
Dialing a Single Socket
To establish a connection with multiple services, create a single gRPC.ClientConn object and pass it to the New*Client() functions for each service. These functions will share the same connection, allowing you to access all services through a single socket.
<code class="go">// ... grpc service server implementation ... func main() { cc, err := grpc.Dial("localhost:6000", grpc.WithInsecure()) if err != nil { log.Fatal(err) } c1 := pb.NewSubscriberServiceClient(cc) c2 := pb.NewDropperServiceClient(cc) // ... }</code>
Avoiding Code Duplication
Instead of creating separate structs for each service, you can use a single struct that implements the client interfaces for both services. This technique reduces code duplication and simplifies your code.
<code class="go">// ... grpc service server implementation ... type SubscriberDropper struct { pb.SubscriberServiceClient pb.DropperServiceClient } func main() { // ... as above ... sd := &SubscriberDropper{c1, c2} // ... }</code>
By leveraging the ability to multiplex services over a single connection, you can simplify your code, enhance efficiency, and reduce resource overhead.
The above is the detailed content of How Can I Access Multiple gRPC Services Over a Single Connection?. For more information, please follow other related articles on the PHP Chinese website!
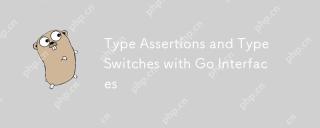
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
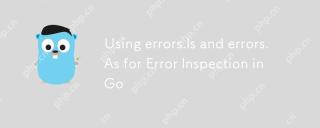
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
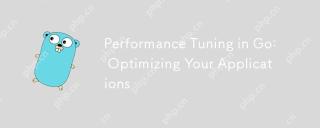
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement
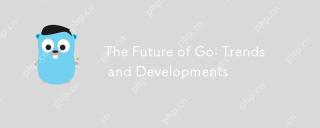
Go'sfutureisbrightwithtrendslikeimprovedtooling,generics,cloud-nativeadoption,performanceenhancements,andWebAssemblyintegration,butchallengesincludemaintainingsimplicityandimprovingerrorhandling.
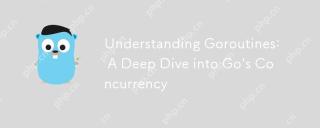
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
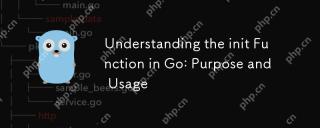
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
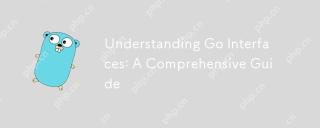
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
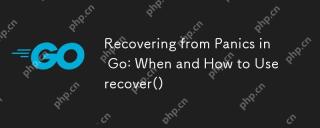
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
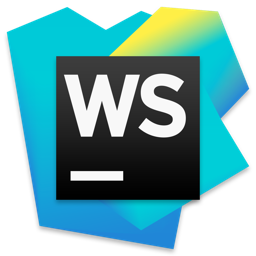
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
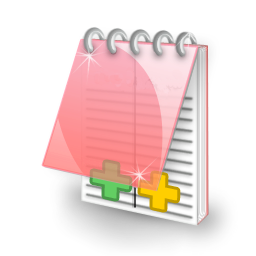
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
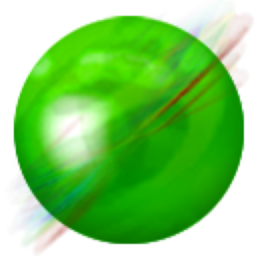
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
