Unmarshaling Nested JSON Without Structural Knowledge
Problem Statement
When dealing with nested JSON data, it can be challenging to determine the appropriate struct to unmarshal into. This is especially true for data stored in a key-value store, where the structure of the JSON data can vary widely.
Question 1: Avoiding Repeated Unmarshaling
While repeated unmarshaling may incur some performance overhead, it is not necessarily something to avoid unless it becomes a significant bottleneck.
Question 2: Determining the Struct Type
There are two main methods to determine the struct type:
Method 1: Unmarshaling to an Interface
- Unmarshal the json.RawMessage to a map[string]interface{} using json.Unmarshal(*objmap["foo"], &myMap).
- Check the type of the nested JSON data in myMap. It will reveal the actual type of the data (e.g., Baz, Bar).
Method 2: Using Regular Expressions
- Create a regular expression to extract the type field from the nested JSON data.
- Use the regexp.FindString function to extract the type.
Once the struct type is determined, you can then unmarshal the data:
varbazorbar interface{} if err := json.Unmarshal(*objmap["foo"], &bazorbar); err !=nil{ return err } switch v := bazorbar.(type) { case map[string]*json.RawMessage: // If the data is a nested JSON object, further unmarshaling is needed. result, err := unmarshalNested(v["nested_data"]) if err != nil return err foo.Nested_Data = result case []interface{}: // If the data is an array of JSON objects, process each element. for _, item := range v { result, err := unmarshalNested(item.(map[string]interface{})) if err != nil return err foo.Nested_Array = append(foo.Nested_Array, result) } }
Example Code:
// Unmarshals nested JSON data. func unmarshalNested(data map[string]interface{}) (interface{}, error) { type_expr := regexp.MustCompile(`"type":\s*"([a-zA-Z]+)"`) matches := type_expr.FindStringSubmatch(data["foo"].(string)) if len(matches) == 2 { switch matches[1] { case "Baz": var baz Baz if err := json.Unmarshal([]byte(data["foo"].(string)), &baz); err != nil { return nil, err } return baz, nil case "Bar": var bar Bar if err := json.Unmarshal([]byte(data["foo"].(string)), &bar); err != nil { return nil, err } return bar, nil } } return nil, errors.New("cannot determine type") }
The above is the detailed content of How to Unmarshal Nested JSON Data Without Knowing Its Structure?. For more information, please follow other related articles on the PHP Chinese website!
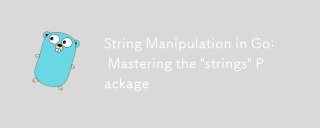
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
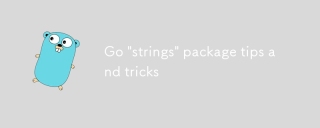
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
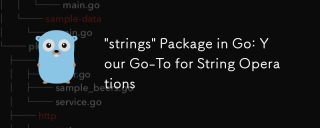
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
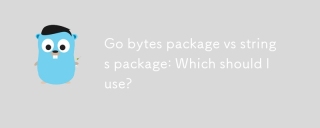
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
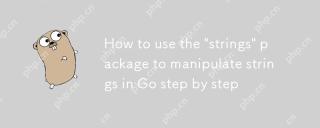
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
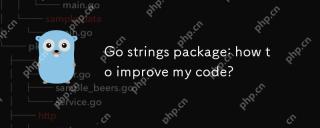
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
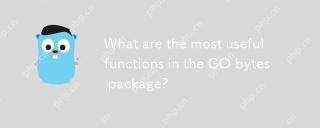
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
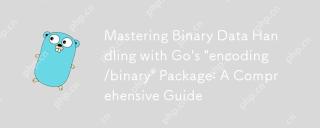
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
