Understanding the Copy Function
The "copy" function in Go is used to transfer elements from a source slice to a destination slice. Let's delve into its behavior and usage.
Basic Operation
According to the documentation, the "copy" function copies elements from the source slice into the destination slice. It takes two arguments:
- dst: Destination slice to which elements will be copied.
- src: Source slice from which elements are copied.
The function returns an integer representing the number of elements copied.
Overlapping Slices
One important feature of "copy" is its ability to handle overlapping slices. If the source and destination slices share the same underlying array, the function successfully completes the copy operation.
Determining Copy Count
The number of elements copied is determined by the minimum length between the source and destination slices. If the source slice has fewer elements than the destination slice, only the number of elements in the source will be copied. Conversely, if the destination slice has fewer elements than the source slice, only the number of elements that fit in the destination will be copied.
Example Usage
Consider the following example where we copy elements from a source slice src into a destination slice dst:
<code class="go">package main import "fmt" func main() { src := []int{10, 11, 12, 13, 14} dst := []int{0, 1, 2, 3, 4} n := copy(dst, src) fmt.Println("n =", n, "src =", src, "dst =", dst) }</code>
Output:
n = 5 src = [10 11 12 13 14] dst = [10 11 12 13 14]
In this example, five elements are copied from src into dst. Both slices have a capacity of five, which is sufficient to hold all elements from the source slice.
Special Case: Copying Bytes from a String
"copy" can also be used to copy bytes from a string (which is essentially a slice of bytes) to a destination slice of bytes. This feature allows for easy manipulation of strings.
Summary
The "copy" function is a versatile tool for transferring elements between slices, considering slice lengths and handling overlapping slices. Understanding its behavior is crucial for effective slice manipulation in Go programs.
The above is the detailed content of How does the \'copy\' function in Go handle overlapping slices?. For more information, please follow other related articles on the PHP Chinese website!
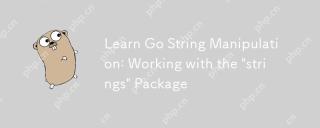
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
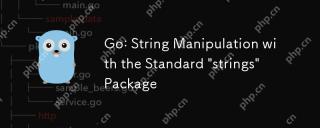
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
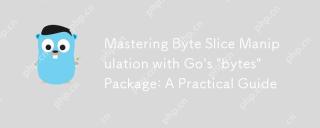
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
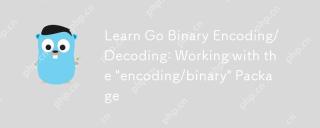
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
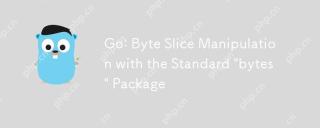
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
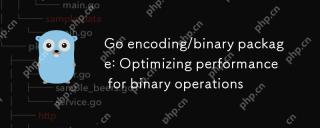
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
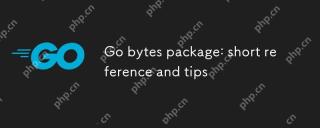
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
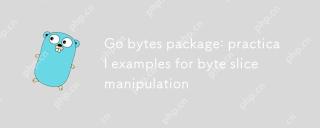
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
