


Why am I getting the \'Invalid hook call\' error in React and how can I fix it?
Invalid Hook Call in React: Understanding the Issue and Resolution
The error "Invalid hook call. Hooks can only be called inside of the body of a function component" arises when hooks are invoked improperly within a React application. This error typically occurs for one of the following reasons:
- Mismatching React and Renderer Versions: Ensure the versions of React and the renderer (such as React DOM) are aligned.
- Breaking the Rules of Hooks: Adhere to the guidelines for using hooks in function components, such as calling hooks only inside functional components and avoiding calling them more than once per component.
- Multiple Copies of React: Avoid having multiple instances of React in a single application, which can lead to conflicts.
In the provided code snippet, the error occurs because hooks (specifically the useState hook) are being employed within a class component. This is incorrect as hooks are exclusively designed for use in functional components.
To resolve the issue, the code should be rewritten as a function component, as seen below:
<code class="javascript">import React, { useState } from 'react'; import { makeStyles } from '@material-ui/core/styles'; import Table from '@material-ui/core/Table'; import TableBody from '@material-ui/core/TableBody'; import TableCell from '@material-ui/core/TableCell'; import TableHead from '@material-ui/core/TableHead'; import TableRow from '@material-ui/core/TableRow'; import Paper from '@material-ui/core/Paper'; const useStyles = makeStyles(theme => ({ root: { width: '100%', marginTop: theme.spacing(3), overflowX: 'auto' }, table: { minWidth: 650 } })); const Allowance = () => { const classes = useStyles(); const [allowances, setAllowances] = useState([]); const fetchAllowances = async () => { const res = await fetch('http://127.0.0.1:8000/allowances'); const data = await res.json(); setAllowances(data); }; useEffect(() => { fetchAllowances(); }, []); return ( <paper classname="{classes.root}"> <table classname="{classes.table}"> <tablehead> <tablerow> <tablecell>Allow ID</tablecell> <tablecell align="right">Description</tablecell> <tablecell align="right">Allow Amount</tablecell> <tablecell align="right">AllowType</tablecell> </tablerow> </tablehead> <tablebody> {allowances.map(row => ( <tablerow key="{row.id}"> <tablecell component="th" scope="row"> {row.AllowID} </tablecell> <tablecell align="right">{row.AllowDesc}</tablecell> <tablecell align="right">{row.AllowAmt}</tablecell> <tablecell align="right">{row.AllowType}</tablecell> </tablerow> ))} </tablebody> </table> </paper> ); }; export default Allowance;</code>
By converting the component to a functional component, the hook can be correctly utilized, resolving the "Invalid hook call" error.
The above is the detailed content of Why am I getting the \'Invalid hook call\' error in React and how can I fix it?. For more information, please follow other related articles on the PHP Chinese website!
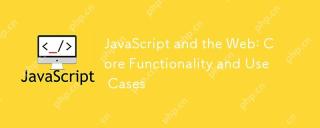
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
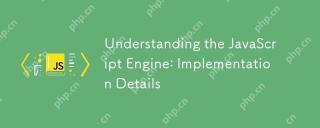
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
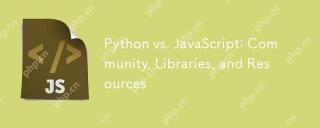
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
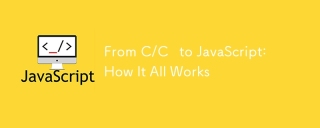
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
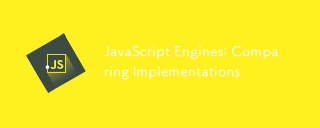
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
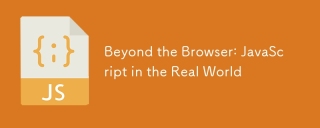
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
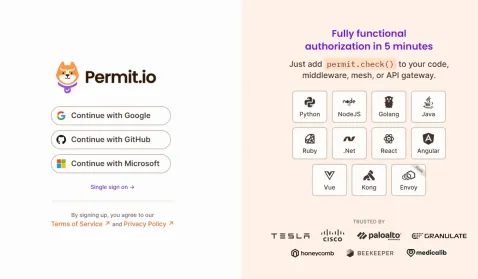
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
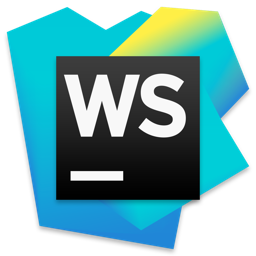
WebStorm Mac version
Useful JavaScript development tools
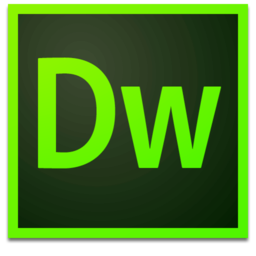
Dreamweaver Mac version
Visual web development tools
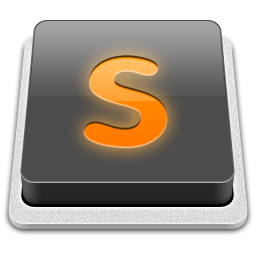
SublimeText3 Mac version
God-level code editing software (SublimeText3)