


How can I pass function pointers to C code in Go 1.6 given the new restrictions?
Passing Function Pointers to C Code Using cgo: Addressing Runtime Changes in Go 1.6
Introduction
Go 1.6 introduced modifications to the rules for passing pointers to C code via cgo. As a result, previously viable methods for calling dynamic Go callbacks from C code are no longer functional. This article explores the updated guidelines and demonstrates how to effectively pass function pointers to C code in the current Go ecosystem.
Passing Function Pointers in Go 1.6
Starting from Go 1.6, the following rule applies:
Go code may pass a Go pointer to C provided that the Go memory to which it points does not contain any Go pointers.
This restriction stems from runtime checks that monitor for violations and provoke crashes when detected. Setting the environment variable GODEBUG=cgocheck=0 temporarily disables these checks, but their removal in future Go versions is possible.
Consequences and Solutions
The new rule prohibits passing pointers to C code if the pointed-to memory holds Go function/method pointers. Several approaches exist to circumvent this limitation:
Synchronization and ID Correspondence
One strategy involves maintaining a synchronized data structure that maps IDs to actual pointers. By passing IDs to C code instead of direct pointers, you can bypass the restriction while still achieving the desired functionality.
Examplar Code
The following code snippet illustrates how to implement this approach:
<code class="go">import ( "fmt" "sync" ) var mu sync.Mutex var index int var fns = make(map[int]func(C.int)) func register(fn func(C.int)) int { mu.Lock() defer mu.Unlock() index++ for fns[index] != nil { index++ } fns[index] = fn return index } func lookup(i int) func(C.int) { mu.Lock() defer mu.Unlock() return fns[i] }</code>
This code defines a registry system that assigns unique IDs to Go functions and stores the mappings in a thread-safe manner. When calling C code, you can pass the ID instead of the function pointer directly.
Conclusion
The changes in Go 1.6 necessitate careful consideration when passing function pointers to C code. By adopting synchronization techniques like the one presented above, you can effectively overcome the new restrictions and ensure compatibility with the latest versions of Go.
The above is the detailed content of How can I pass function pointers to C code in Go 1.6 given the new restrictions?. For more information, please follow other related articles on the PHP Chinese website!
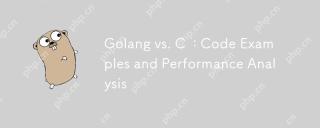
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
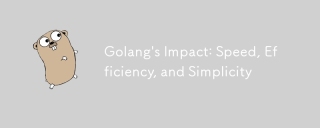
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
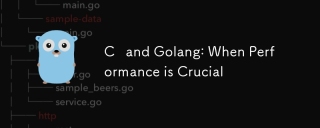
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
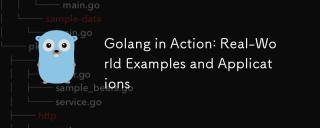
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
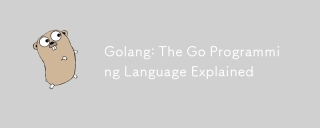
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
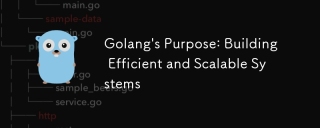
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
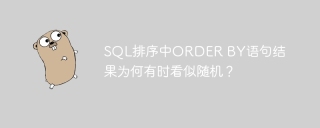
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
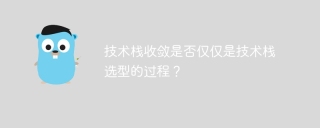
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
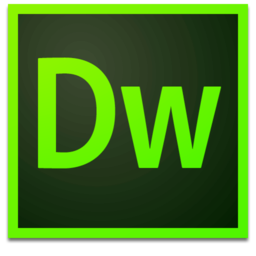
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.