Unzipping Files Asynchronously in a Web Browser
Unzipping Zipped Files for Display in a Web Browser
To display OpenOffice files (.odt and .odp) in a web browser, one needs to unzip them first. While inflate.js may not suffice for this task, there exists a robust JavaScript solution to unzip ZIP files asynchronously.
JavaScript Unzipper
A JavaScript unzipper has been developed, incorporating elements from Andy G.P. Na's binary file reader and Notmasteryet's RFC1951 inflate logic. The ZipFile class handles the core functionality.
Implementation in JavaScript
The following JavaScript code demonstrates the usage of the unzipper:
// Instantiate a ZipFile and provide a callback for when the ZIP is read. zipFile = new ZipFile(url, doneReading); // The doneReading callback is triggered when the ZIP is read and handles the extraction of entries. function doneReading(zip) { extractEntries(zip); } // Extract entries from the ZIP file. function extractEntries(zip) { for (var i = 0; i <a>" + entry.name + "</a>\n<div>"; // Create a unique ID for the accordion panel and inject the extracted content into it. var randomId = "id-" + Math.floor((Math.random() * 1000000000)); entryInfo += "<span class="inputDiv"><h4 id="Content">Content:</h4> <span id='" + randomId + "'></span></span> </div>\n"; $("#report").append(entryInfo); // Asynchronously extract the entry and pass a callback. entry.extract(extractCb(randomId)); } } // Extract callback function to add the extracted content to an accordion on the page. function extractCb(id) { return (function(entryName, entryText){ var content = entryText.replace(new RegExp( "\n", "g" ), "<br>"); $("#"+id).html(content); $('#report').accordion('destroy'); $('#report').accordion({collapsible:true, active:false}); }); }
Limitations
While the JavaScript unzipper fulfills its purpose, it faces certain limitations:
- Performance: Decompression can be slow compared to compiled programs, especially with large ZIP files.
- Memory Usage: The unzipper reads the entire ZIP into memory, which can strain system resources with large files.
- Limited Functionality: It does not support all ZIP features, such as encryption, Zip64, and UTF-8 encoded filenames.
The above is the detailed content of How Can I Unzip Files Asynchronously in a Web Browser Using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
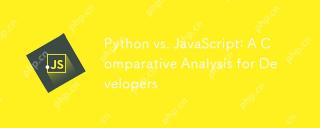
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
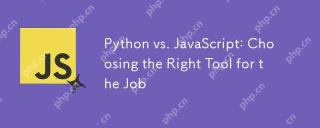
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
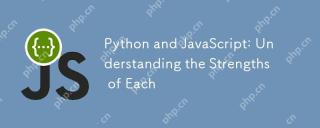
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
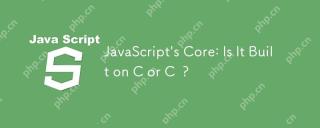
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
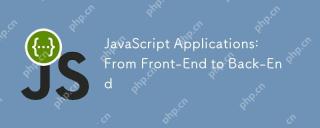
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
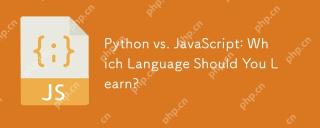
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
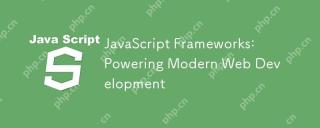
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
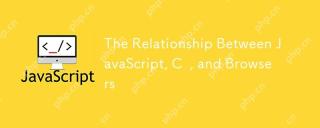
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment
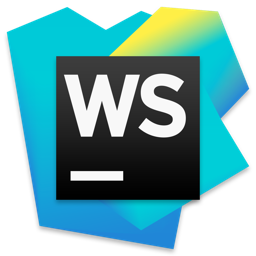
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Chinese version
Chinese version, very easy to use
