


Can I Resolve This with Pure MySQL? (Joining on ; Separated Values in a Column)
Problem:
You have data stored in two tables, with one column in the first table listing multiple values separated by semicolons. You need to perform an inner join based on these separated values, but you don't have a linking table and cannot use any external programming languages.
Discussion:
The challenge lies in converting the semicolon-separated list into separate rows, making it possible to join with the second table. This can be achieved using a technique called "deriving" or "normalizing" the data.
Solution:
1. Create an Integerseries Table:
To normalize the data, you first need a table that contains a range of numbers. In this case, you could create an integerseries table with IDs from 1 to the maximum number of elements you expect in the semicolon-separated list.
2. Use JOIN and Subqueries:
Once you have the integerseries table, perform the following query:
<code class="sql">SELECT user_resource.user, resource.data FROM user_resource JOIN integerseries AS isequence ON isequence.id <p><strong>Explanation:</strong></p> <ul> <li>This query joins the user_resource table with the integerseries table to generate a set of rows for each user and each element in the corresponding resources column.</li> <li>The COUNT_IN_SET function counts the number of elements in the resources column, and the VALUE_IN_SET function extracts a specific element based on the isequence.id.</li> <li>The final join with the resource table matches the extracted element with the ID to retrieve the corresponding data.</li> </ul> <p><strong>Additional Functions (Optional):</strong></p> <p>The query uses two custom functions, COUNT_IN_SET and VALUE_IN_SET, which can be defined as follows:</p> <pre class="brush:php;toolbar:false"><code class="sql">-- Function to count the number of delimited items in a string DELIMITER $$ DROP FUNCTION IF EXISTS `COUNT_IN_SET`$$ CREATE FUNCTION `COUNT_IN_SET`(haystack VARCHAR(1024), delim CHAR(1) ) RETURNS INTEGER BEGIN RETURN CHAR_LENGTH(haystack) - CHAR_LENGTH( REPLACE(haystack, delim, '')) + 1; END$$ DELIMITER ; -- Function to get the value at a specific index in a delimited string DELIMITER $$ DROP FUNCTION IF EXISTS `VALUE_IN_SET`$$ CREATE FUNCTION `VALUE_IN_SET`(haystack VARCHAR(1024), delim CHAR(1), which INTEGER ) RETURNS VARCHAR(255) CHARSET utf8 COLLATE utf8_unicode_ci BEGIN RETURN SUBSTRING_INDEX(SUBSTRING_INDEX(haystack, delim, which), delim, -1); END$$ DELIMITER ;</code>
These functions provide a generic way to manipulate delimited strings within SQL queries.
Example Tables and Data:
<code class="sql">-- Integerseries table CREATE TABLE `integerseries` ( `id` int(11) NOT NULL AUTO_INCREMENT, PRIMARY KEY (`id`) ); -- Resource table CREATE TABLE `resource` ( `id` int(11) NOT NULL, `data` varchar(250) DEFAULT NULL, PRIMARY KEY (`id`) ); -- Data for resource table INSERT INTO `resource` (`id`, `data`) VALUES (1, 'abcde'), (2, 'qwerty'), (3, 'azerty'); -- User_resource table CREATE TABLE `user_resource` ( `user` varchar(50) NOT NULL, `resources` varchar(250) DEFAULT NULL, PRIMARY KEY (`user`) ); -- Data for user_resource table INSERT INTO `user_resource` (`user`, `resources`) VALUES ('sampleuser', '1;2;3'), ('stacky', '2'), ('testuser', '1;3');</code>
Output:
Executing the query on the sample data will produce the following output:
+----------+-------+ | user | data | +----------+-------+ | sampleuser | abcde | | sampleuser | qwerty | | sampleuser | azerty | | stacky | qwerty | | testuser | abcde | | testuser | azerty | +----------+-------+
The above is the detailed content of Can I Join Tables on Semicolon-Separated Values in MySQL Without External Tools?. For more information, please follow other related articles on the PHP Chinese website!
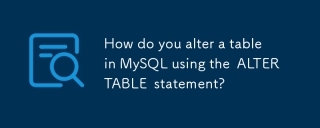
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
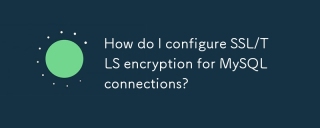
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
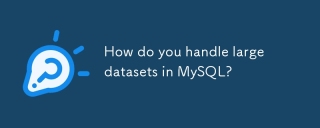
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
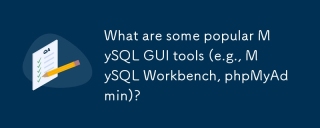
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
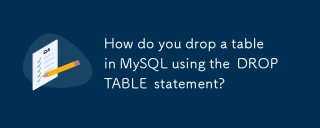
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
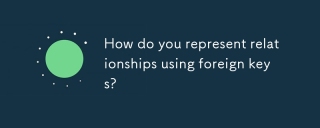
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
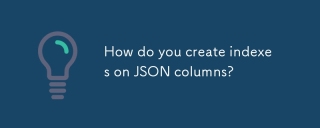
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
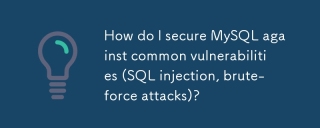
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
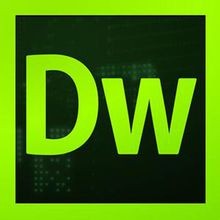
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
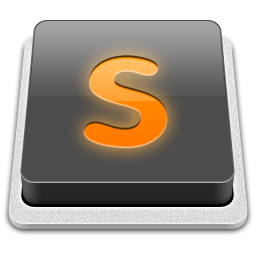
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
