


PHP: How to Obtain All Possible Combinations of a One-Dimensional Array
In this article, we will dive into a method to retrieve all possible combinations of elements within a one-dimensional array in PHP. In contrast to other solutions, this approach ensures that both combinations and permutations are considered.
Problem Statement
Given an array of strings or numbers, the goal is to generate all possible combinations of elements, taking into account different arrangements. For instance, 'Alpha Beta' and 'Beta Alpha' are distinct combinations that should be included in the output.
PHP Solution
The provided PHP solution employs a recursive depth-first search algorithm:
<code class="php"><?php $array = array('Alpha', 'Beta', 'Gamma', 'Sigma'); function depth_picker($arr, $temp_string, &$collect) { if ($temp_string != "") $collect []= $temp_string; for ($i=0, $iMax = sizeof($arr); $i < $iMax; $i++) { $arrcopy = $arr; $elem = array_splice($arrcopy, $i, 1); // removes and returns the i'th element if (sizeof($arrcopy) > 0) { depth_picker($arrcopy, $temp_string ." " . $elem[0], $collect); } else { $collect []= $temp_string. " " . $elem[0]; } } } $collect = array(); depth_picker($array, "", $collect); print_r($collect); ?></code>
Breakdown of the Solution
- The depth_picker function is invoked with the initial array, an empty string, and an empty array reference.
- It recursively loops through the array, adding each element to the end of the current result string.
- Whenever the temp string has elements, it is added to the result array.
- If there are still elements left in the input array, the function calls itself with the updated array and temp string.
- If there are no more elements left, the temp string containing the complete combination is added to the collect array.
Output
The code provided produces the following output, which contains all combinations and permutations of the elements in the given array:
Array ( [0] => Alpha [1] => Alpha Beta [2] => Alpha Beta Gamma [3] => Alpha Beta Gamma Sigma [4] => Alpha Beta Sigma [5] => Alpha Beta Sigma Gamma [6] => Alpha Gamma [7] => Alpha Gamma Beta [8] => Alpha Gamma Beta Sigma [9] => Alpha Gamma Sigma [10] => Alpha Gamma Sigma Beta [11] => Alpha Sigma [12] => Alpha Sigma Beta [13] => Alpha Sigma Beta Gamma [14] => Alpha Sigma Gamma [15] => Alpha Sigma Gamma Beta [16] => Beta [17] => Beta Alpha [18] => Beta Alpha Gamma [19] => Beta Alpha Gamma Sigma [20] => Beta Alpha Sigma [21] => Beta Alpha Sigma Gamma [22] => Beta Gamma [23] => Beta Gamma Alpha [24] => Beta Gamma Alpha Sigma [25] => Beta Gamma Sigma [26] => Beta Gamma Sigma Alpha [27] => Beta Sigma [28] => Beta Sigma Alpha [29] => Beta Sigma Alpha Gamma [30] => Beta Sigma Gamma [31] => Beta Sigma Gamma Alpha [32] => Gamma [33] => Gamma Alpha [34] => Gamma Alpha Beta [35] => Gamma Alpha Beta Sigma [36] => Gamma Alpha Sigma [37] => Gamma Alpha Sigma Beta [38] => Gamma Beta [39] => Gamma Beta Alpha [40] => Gamma Beta Alpha Sigma [41] => Gamma Beta Sigma [42] => Gamma Beta Sigma Alpha [43] => Gamma Sigma [44] => Gamma Sigma Alpha [45] => Gamma Sigma Alpha Beta [46] => Gamma Sigma Beta [47] => Gamma Sigma Beta Alpha [48] => Sigma [49] => Sigma Alpha [50] => Sigma Alpha Beta [51] => Sigma Alpha Beta Gamma [52] => Sigma Alpha Gamma [53] => Sigma Alpha Gamma Beta [54] => Sigma Beta [55] => Sigma Beta Alpha [56] => Sigma Beta Alpha Gamma [57] => Sigma Beta Gamma [58] => Sigma Beta Gamma Alpha [59] => Sigma Gamma [60] => Sigma Gamma Alpha [61] => Sigma Gamma Alpha Beta [62] => Sigma Gamma Beta [63] => Sigma Gamma Beta Alpha )
The above is the detailed content of How can I generate all possible combinations and permutations of elements within a one-dimensional array in PHP?. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
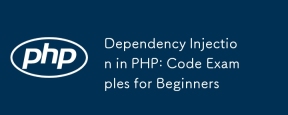
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
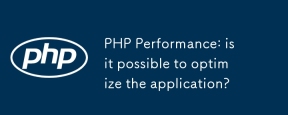
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
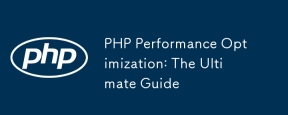
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
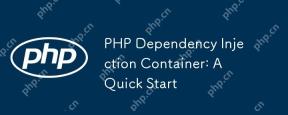
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
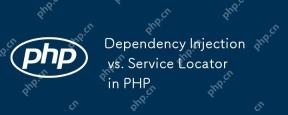
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
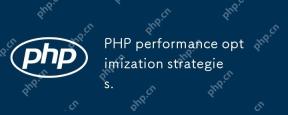
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
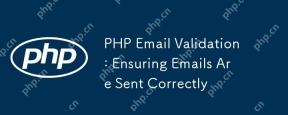
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
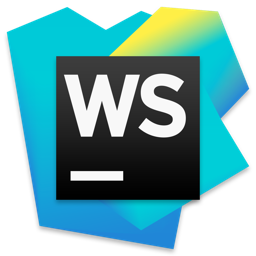
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
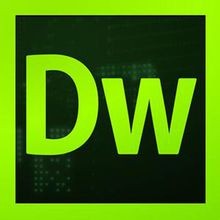
Dreamweaver CS6
Visual web development tools
