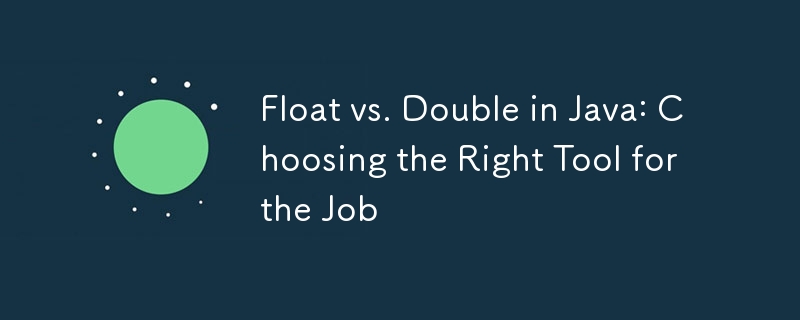
When working with decimal numbers in Java, you have two main options: float and double. While both represent floating-point values, they differ in precision, memory usage, and ideal use cases.
The Differences
- Precision: Float offers approximately 7 decimal digits of precision, while double provides around 15 decimal digits.
- Memory Usage: Float takes up 4 bytes of memory, while double occupies 8 bytes.
- Speed: Float calculations are slightly faster than double, but this difference is often negligible on modern hardware.
- Range of Values: Double can represent a much wider range of values than float.
When to Use float
Choose float when:
- Memory is a Concern: If you're dealing with a large amount of floating-point data (e.g., in scientific simulations or 3D graphics), using float can save significant memory.
- Precision Isn't Critical: When you don't need extreme accuracy, float is often sufficient. Use it for representing pixel coordinates in graphics, approximate measurements in simulations, or temperatures where minor rounding errors are acceptable.
Example of float
float temperatureCelsius = 25.5f;
float gravityMetersPerSecondSquared = 9.8f;
When to Use double
Choose double when:
- Precision Matters: For calculations where accuracy is paramount, double is the way to go. It's suitable for financial calculations, scientific simulations requiring high precision, and any situation where rounding errors can lead to significant problems.
- Large Range of Values: If you need to represent extremely large or small numbers, double offers a wider range than float.
Example of double
double pi = 3.14159265358979323846;
double avogadrosNumber = 6.02214076e23;
Rule of Thumb
If you're unsure, err on the side of using double. Its increased precision and range will often be more beneficial than the slight memory savings offered by float.
Key Takeaway:
The choice between float and double boils down to balancing precision and memory usage. By understanding their strengths and weaknesses, you can make informed decisions for your specific applications.
The above is the detailed content of Float vs. Double in Java: Choosing the Right Tool for the Job. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn