


How can I achieve mutual exclusion in concurrent goroutines using WaitGroup?
Mutual Exclusion of Concurrent Goroutines Using WaitGroup
In your code, you have three concurrent goroutines that need to execute without interference from each other during specific sections of their code. This concept is known as mutual exclusion, and it ensures that only one goroutine can execute a critical section of code at a time.
To achieve mutual exclusion using WaitGroup, you can follow these steps:
- Create a Mutex for Each Concurrent Goroutine: A mutex is a locking mechanism that allows only one goroutine to acquire a lock at a time. Create a separate mutex for each goroutine that needs to execute its critical section exclusively.
- Acquire the Mutex Before Entering the Critical Section: Before executing the critical section of code in each goroutine, call the Lock() method on the corresponding mutex. This operation will block the goroutine until the mutex is acquired.
- Release the Mutex After Leaving the Critical Section: After completing execution of the critical section, release the mutex by calling the Unlock() method. This allows other goroutines to acquire the mutex and enter their critical sections.
- Use WaitGroup to Wait for Completion: Create a WaitGroup to track the completion of all goroutines. Each goroutine should call the Done() method of the WaitGroup after completing its execution. The main goroutine should wait for all goroutines to complete using the Wait() method of the WaitGroup.
Here's an example that implements the above steps:
<code class="go">package main import ( "fmt" "sync" ) var ( mutex1 sync.Mutex mutex2 sync.Mutex mutex3 sync.Mutex wg sync.WaitGroup ) func Routine1() { mutex1.Lock() defer mutex1.Unlock() // Do something for i := 0; i <p>In this example, the critical section of each goroutine is the loop where it executes fmt.Println("value of z"). The mutexes ensure that only one goroutine can execute this section at a time. The WaitGroup ensures that the main goroutine waits for all goroutines to complete before exiting.</p></code>
The above is the detailed content of How can I achieve mutual exclusion in concurrent goroutines using WaitGroup?. For more information, please follow other related articles on the PHP Chinese website!
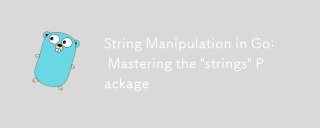
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
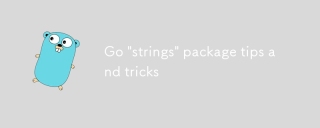
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
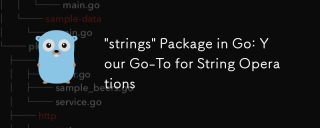
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
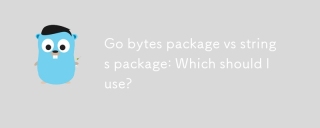
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
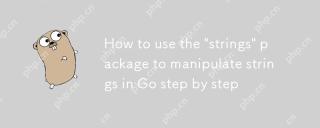
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
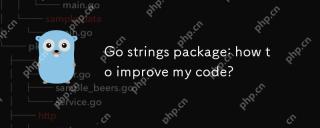
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
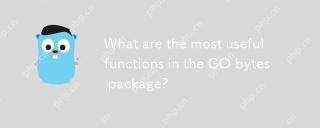
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
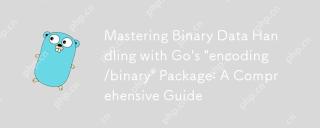
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
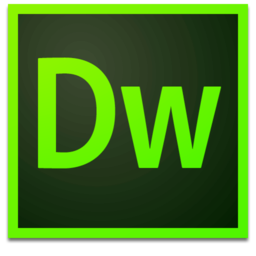
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
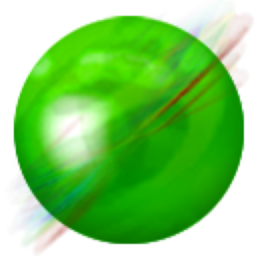
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
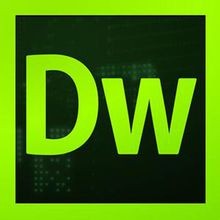
Dreamweaver CS6
Visual web development tools
