Controlling Bandwidth of HTTP Requests in Go
Question:
As a novice to Go, how can I restrict bandwidth consumption during an HTTP GET request?
Background:
Analysis:
You have discovered the mxk/go1/flowcontrol library, which provides low-level control over bandwidth usage. However, integrating it with the http.Get() method requires understanding how to access the underlying reader.
Solution:
While convenient wrappers exist in third-party packages, understanding the fundamentals of bandwidth control can be enlightening. Here's a simple implementation that illustrates the approach:
<code class="go">package main import ( "io" "net/http" "os" "time" ) // Set data chunk size (in bytes) and time interval (in seconds) var datachunk int64 = 500 var timelapse time.Duration = 1 func main() { // Initiate HTTP GET request response, _ := http.Get("http://google.com") // Read response in controlled intervals for range time.Tick(timelapse * time.Second) { // Read a chunk of data into stdout _, err := io.CopyN(os.Stdout, response.Body, datachunk) if err != nil { break } } }</code>
Explanation:
- __io.CopyN()__: Reads a specified number of bytes (datachunk) from the response body at controlled intervals (timelapse).
- __os.Stdout__: Represents the standard output, where the chunks are printed.
This code demonstrates how to manually limit bandwidth consumption while maintaining the core functionality of the http.Get() method.
The above is the detailed content of How to Limit Bandwidth Usage During an HTTP GET Request in Go?. For more information, please follow other related articles on the PHP Chinese website!
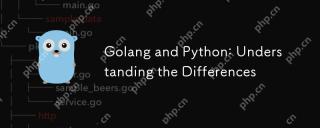
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
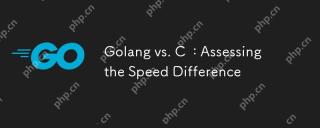
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
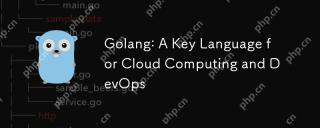
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
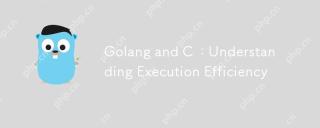
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
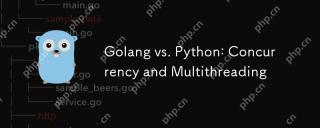
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
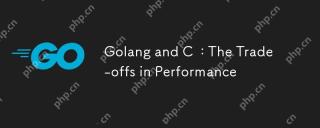
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
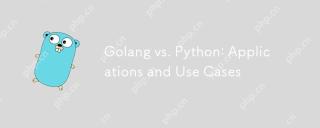
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
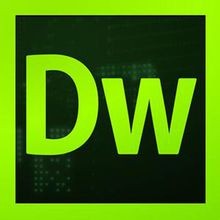
Dreamweaver CS6
Visual web development tools
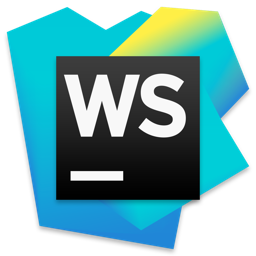
WebStorm Mac version
Useful JavaScript development tools
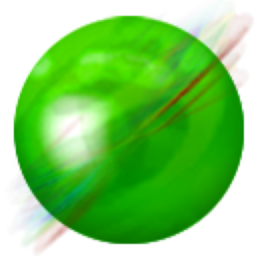
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor