Object Serialization in C Using the Factory Pattern
In C , serialization involves converting an object's state into a stream for storage or transmission and later reconstructing the object from the stream. A common approach is using class IDs for serialization and deserialization, but this can be considered an antipattern.
Boost Serialization
One alternative is to use a library like Boost Serialization. This library provides a comprehensive framework for object serialization, handling the low-level details and offering a user-friendly interface.
Factory Pattern with Registered Classes
Another approach is to use the factory pattern with registered classes. Here's how it works:
- Create a factory class that stores a map between keys (class IDs) and function pointers called "creators."
- Register classes with the factory by calling the factory's registerClass() method, passing the class ID and a creator function for that class.
- Implement the creator function as a static member function that creates an instance of the respective class.
- To create an object from a class, call the factory's createObject() method with the corresponding class ID.
Code Example
The following C code demonstrates an implementation of the object factory:
<code class="cpp">template<typename k typename t> class Factory { typedef T *(*CreateObjectFunc)(); std::map<k createobjectfunc> mObjectCreator; template<typename s> static T* createObject(){ return new S(); } public: template<typename s> void registerClass(K id){ mObjectCreator.insert( std::make_pair<k>(id, &createObject<s> ) ); } bool hasClass(K id){ return mObjectCreator.find(id) != mObjectCreator.end(); } T* createObject(K id){ return ((*mObjectCreator[id])(); } };</s></k></typename></typename></k></typename></code>
The above is the detailed content of How can the Factory Pattern be leveraged for Object Serialization in C ?. For more information, please follow other related articles on the PHP Chinese website!
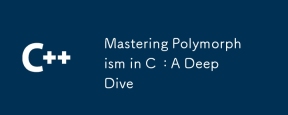
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
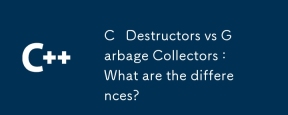
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
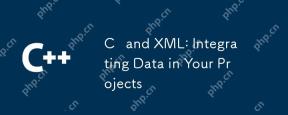
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
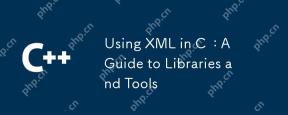
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
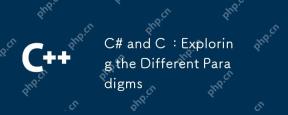
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
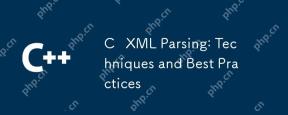
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
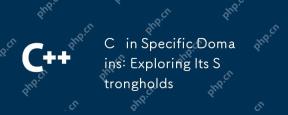
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
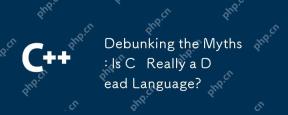
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
