Capacity of Slices After Dropping Items
When working with slices in Go, it's important to understand how their capacity changes based on the type of modification made. To illustrate this, let's examine a code snippet and its output:
package main import "fmt" func main() { s := []int{2, 3, 5, 7, 11, 13} printSlice(s) // Drop its last two values s = s[:len(s)-2] printSlice(s) // Drop its first two values. s = s[2:] printSlice(s) } func printSlice(s []int) { fmt.Printf("len=%d cap=%d %v\n", len(s), cap(s), s) }
Output:
len=6 cap=6 [2 3 5 7 11 13] len=4 cap=6 [2 3 5 7] len=2 cap=4 [5 7]
Why Different Capacities After Dropping Items?
Notice that when we drop the last two items from the slice (s = s[:len(s)-2]), the capacity remains unchanged at 6, while dropping the first two items (s = s[2:]) reduces the capacity to 4.
The reason for this difference lies in how slices are implemented in Go. Slices are essentially a view into an underlying array. Dropping the last two values adjusts the slice's length, which is the number of elements the slice points to, but it does not affect the capacity, which is the size of the underlying array.
However, dropping the first two values results in a new underlying array being created to hold the reduced slice. This is because the elements in the original underlying array are shifted down two places to fill the gap, and a new array is necessary to accommodate the shifted elements.
The above is the detailed content of How Does Dropping Elements from a Go Slice Affect Its Capacity?. For more information, please follow other related articles on the PHP Chinese website!
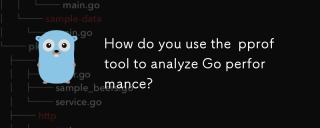
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
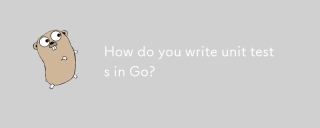
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
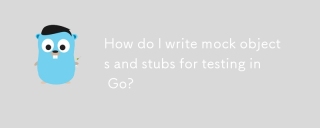
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
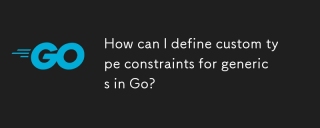
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
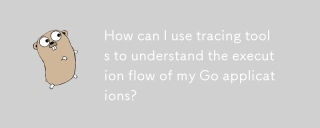
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
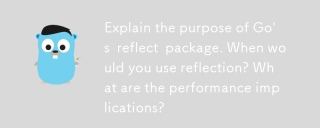
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
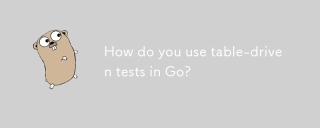
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
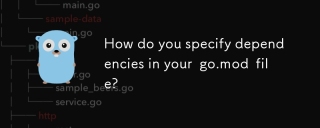
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
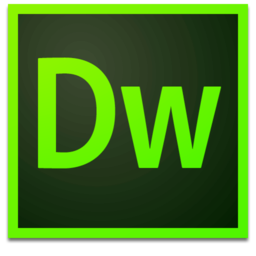
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
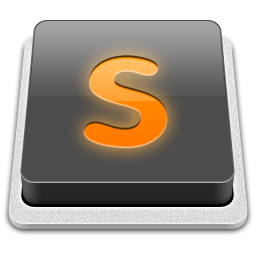
SublimeText3 Mac version
God-level code editing software (SublimeText3)
