


Can't Find a Way to Color the Mandelbrot-Set the Way I'm Aiming For
In this post, the individual aims to generate colorful Mandelbrot sets while maintaining detail during zooming. However, they encounter limitations in their current approach. Let's delve into the issue and provide a detailed solution:
The primary concern is how to achieve beautiful colors throughout the zooming process while ensuring that the set doesn't become "blurry" or lose its intricate patterns. The problem arises from using the maximum iteration count (max_iterations) as the basis for color calculation. Higher max_iterations result in a broader color spectrum but can lead to visual artifacts, especially during zooming.
To effectively solve this problem, it's necessary to employ two distinct concepts: dynamic max iterations count and fractional escape.
Dynamic Max Iterations Count
Dynamic max iterations count is a technique that adjusts the maximum number of iterations based on the current zoom level. This approach ensures that the algorithm assigns more iterations to regions where intricate details emerge during zooming, providing a more precise representation of the set.
Fractional Escape
Fractional escape refers to the calculation of the escape value as a decimal fraction rather than an integer. This method enables the generation of smoother color gradients, eliminating the visible steps that can occur with integer-based escape calculations.
GLSL Implementation
To implement the aforementioned concepts in GLSL, consider using the following code snippet:
<code class="glsl">// Calculate the escape value as a fractional part mu = m + frac = n + 1 - log(log(abs(Z(n))) / log(2.0)); // Convert the fractional part to fixed point mu *= float(1 N) i = N; if (i <p>In this code, 'mu' represents the fractional escape value, 'm' is the maximum iteration count, 'n' is the current iteration count, and 'sh' is the number of fractional bits used. This modified approach allows for precise color calculation based on the fractional escape value.</p> <h3 id="Multi-Pass-Recoloring">Multi-Pass Recoloring</h3> <p>To further enhance the color spectrum, consider implementing a multi-pass recoloring technique. This method involves generating multiple images at different max iteration counts and subsequently combining them to create a final image with a broader color range. Here's a simplified explanation of the process:</p> <ol> <li>Render the Mandelbrot set at a low max iteration count, capturing the finer details.</li> <li>Render the same set at a higher max iteration count to obtain a wider color spectrum.</li> <li>Use the lower-resolution image as a mask to blend the colors from the higher-resolution image.</li> </ol> <p>This multi-pass approach helps achieve vibrant and detailed color distributions throughout the zooming process.</p> <p>By incorporating dynamic max iterations count, fractional escape, and multi-pass recoloring into your code, you should be able to create Mandelbrot sets with stunning colors and intricate patterns that persist during zooming.</p></code>
The above is the detailed content of How can I generate colorful Mandelbrot sets that retain their intricate patterns during zooming, avoiding 'blurriness” and artifacts?. For more information, please follow other related articles on the PHP Chinese website!
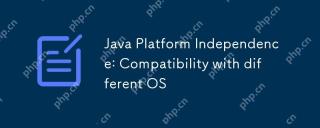
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
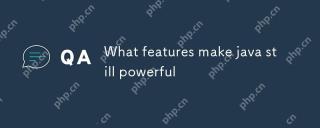
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
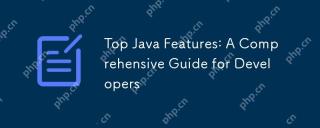
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
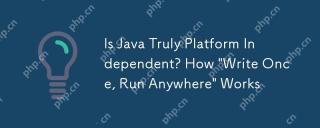
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
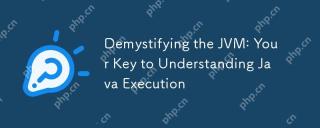
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
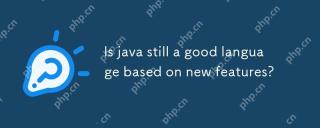
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
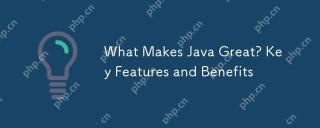
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
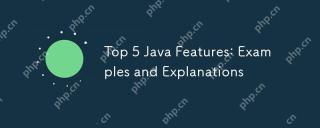
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
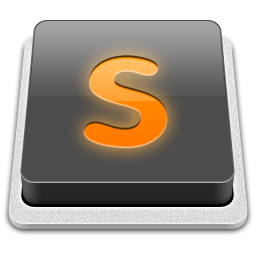
SublimeText3 Mac version
God-level code editing software (SublimeText3)
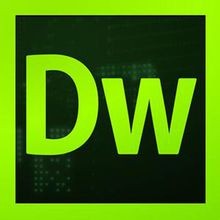
Dreamweaver CS6
Visual web development tools
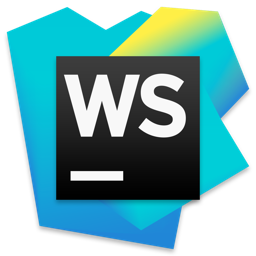
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
