Using setTimeout() in Promise Chains
When working with promises, it's essential to understand how to handle asynchronous operations within a promise chain. In the given code snippet, the intention is to delay the execution of a subsequent request in a promise chain. However, using setTimeout() directly within the .then() handler can lead to issues.
Why setTimeout() Fails
In your code, setTimeout() is used within the .then() handler of the second request. However, this approach breaks the promise chain because the returned value of the .then() handler is not a promise. The setTimeout() callback, which contains the promise you want to chain, is not accessible outside its own context.
Solution Using delay Function
To maintain the promise chain, you can create a separate delay function that returns a promise:
<code class="javascript">function delay(t, val) { return new Promise(resolve => setTimeout(resolve, t, val)); }</code>
Then, use the delay function in your promise chain:
<code class="javascript">return delay(1000).then(function() { return getLinks(globalObj["two"] + ".txt"); });</code>
This returns a promise from the .then() handler, which ensures that the execution of the subsequent request is delayed.
Alternative Using Promise.prototype.delay
Another option is to extend the Promise object with a delay method:
<code class="javascript">Promise.prototype.delay = function(t) { return this.then(function(val) { return delay(t, val); }); }</code>
With this method, you can directly call .delay() on your promises:
<code class="javascript">Promise.resolve("hello").delay(500).then(function(val) { console.log(val); });</code>
Both approaches ensure that the promise chain is maintained correctly and the subsequent request is executed after the specified delay.
The above is the detailed content of How to Properly Incorporate setTimeout() into Promise Chains?. For more information, please follow other related articles on the PHP Chinese website!
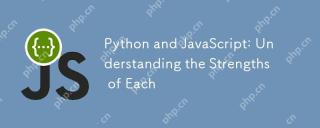
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
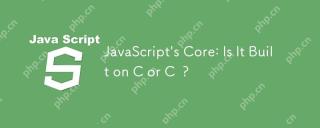
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
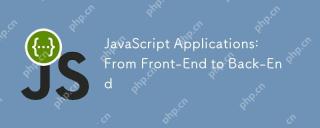
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
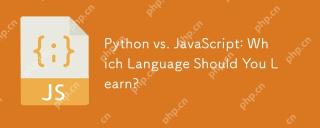
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
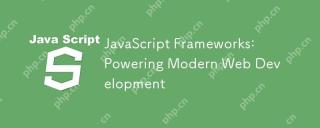
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
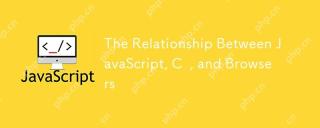
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
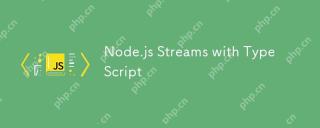
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
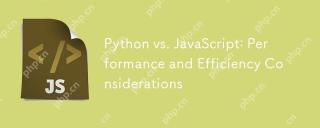
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
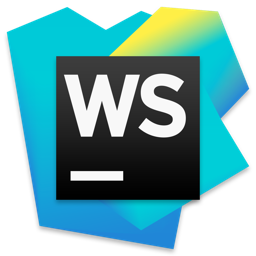
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
