TypeOf Without an Instance: Passing Result to a Function
In Go, it is possible to obtain a "Type" without having an instance of that type. This can be achieved through the use of reflect.TypeOf(), but typically examples involve an instance.
Getting Type Without Instance
To acquire a "Type" without an instance, the trick is to start with a pointer to the type, which can be assigned a "typed" nil. Subsequently, .Elem() can be used to obtain the reflect.Type descriptor of the pointed type, known as the base type.
<code class="go">t := reflect.TypeOf((*int)(nil)).Elem() fmt.Println(t) t = reflect.TypeOf((*http.Request)(nil)).Elem() fmt.Println(t) t = reflect.TypeOf((*os.File)(nil)).Elem() fmt.Println(t)</code>
Example Output:
int http.Request os.File
Passing Type Around
If you need to pass around types and utilize them in switches, create and store them in global variables for reference:
<code class="go">var ( intType = reflect.TypeOf((*int)(nil)) httpRequestType = reflect.TypeOf((*http.Request)(nil)) osFileType = reflect.TypeOf((*os.File)(nil)) int64Type = reflect.TypeOf((*uint64)(nil)) ) func printType(t reflect.Type) { switch t { case intType: fmt.Println("Type: int") case httpRequestType: fmt.Println("Type: http.request") case osFileType: fmt.Println("Type: os.file") case int64Type: fmt.Println("Type: uint64") default: fmt.Println("Type: Other") } } func main() { printType(intType) printType(httpRequestType) printType(osFileType) printType(int64Type) }</code>
Simplified Approach
If you're utilizing the types solely for comparison purposes, consider using constants instead of reflect.Type:
<code class="go">type TypeDesc int const ( typeInt TypeDesc = iota typeHttpRequest typeOsFile typeInt64 ) func printType(t TypeDesc) { switch t { case typeInt: fmt.Println("Type: int") case typeHttpRequest: fmt.Println("Type: http.request") case typeOsFile: fmt.Println("Type: os.file") case typeInt64: fmt.Println("Type: uint64") default: fmt.Println("Type: Other") } } func main() { printType(typeInt) printType(typeHttpRequest) printType(typeOsFile) printType(typeInt64) }</code>
The above is the detailed content of How to Get a Type Without an Instance in Go?. For more information, please follow other related articles on the PHP Chinese website!
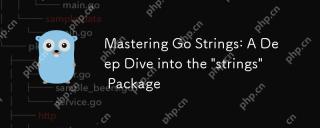
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
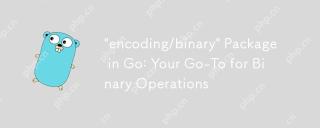
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
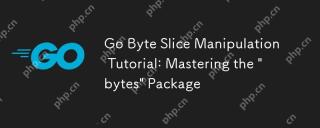
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.
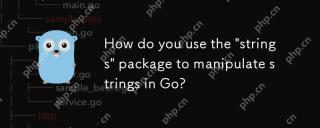
You can use the "strings" package in Go to manipulate strings. 1) Use strings.TrimSpace to remove whitespace characters at both ends of the string. 2) Use strings.Split to split the string into slices according to the specified delimiter. 3) Merge string slices into one string through strings.Join. 4) Use strings.Contains to check whether the string contains a specific substring. 5) Use strings.ReplaceAll to perform global replacement. Pay attention to performance and potential pitfalls when using it.
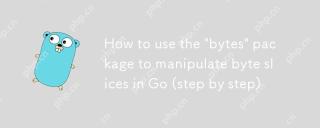
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
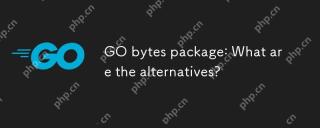
ThealternativestoGo'sbytespackageincludethestringspackage,bufiopackage,andcustomstructs.1)Thestringspackagecanbeusedforbytemanipulationbyconvertingbytestostringsandback.2)Thebufiopackageisidealforhandlinglargestreamsofbytedataefficiently.3)Customstru
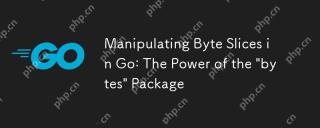
The"bytes"packageinGoisessentialforefficientlymanipulatingbyteslices,crucialforbinarydata,networkprotocols,andfileI/O.ItoffersfunctionslikeIndexforsearching,Bufferforhandlinglargedatasets,Readerforsimulatingstreamreading,andJoinforefficient
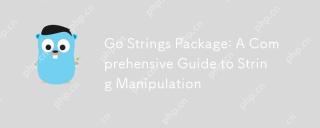
Go'sstringspackageiscrucialforefficientstringmanipulation,offeringtoolslikestrings.Split(),strings.Join(),strings.ReplaceAll(),andstrings.Contains().1)strings.Split()dividesastringintosubstrings;2)strings.Join()combinesslicesintoastring;3)strings.Rep


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
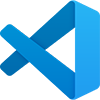
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
