Determining Value Presence in JavaScript: Undefined versus Null
In JavaScript, variables can store various data types, including null and undefined. Distinguishing between these two values is crucial for maintaining code integrity.
Consider the following code snippet:
if (typeof(some_variable) != 'undefined' && some_variable != null) { // Do something with some_variable }
While this pattern is commonly used, it lacks conciseness. A simpler alternative exists:
if (some_variable) { // Do something with some_variable }
However, Firebug may raise errors when using this simplified form for undefined variables. This behavior prompts the question: Are these two methods truly interchangeable?
Defining Variable Presence
The most efficient way to check for null or undefined values is through the following:
if (some_variable == null) { // some_variable is either null or undefined }
This method equates to the following:
if (typeof(some_variable) !== "undefined" && some_variable !== null) {} if (some_variable != null) {}
Note that the simplified form assumes variable declaration; otherwise, a ReferenceError will occur. This assumption is often safe in contexts like checking for optional arguments or properties on existing objects.
Alternatively, the following form is not equivalent:
if (!some_variable) { // some_variable is either null, undefined, 0, NaN, false, or an empty string }
Note: It's generally recommended to use === instead of ==, with the proposed solution being an exception.
The above is the detailed content of Is Checking for Null or Undefined in JavaScript Really That Simple?. For more information, please follow other related articles on the PHP Chinese website!
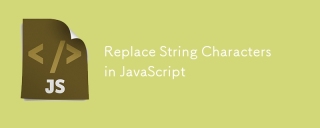
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
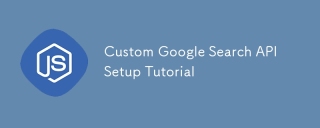
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
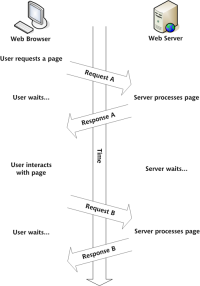
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
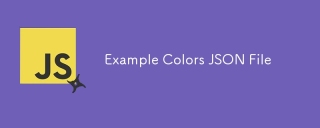
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
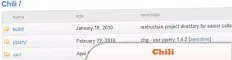
Enhance Your Code Presentation: 10 Syntax Highlighters for Developers Sharing code snippets on your website or blog is a common practice for developers. Choosing the right syntax highlighter can significantly improve readability and visual appeal. T
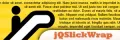
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
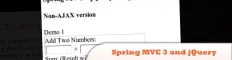
This article presents a curated selection of over 10 tutorials on JavaScript and jQuery Model-View-Controller (MVC) frameworks, perfect for boosting your web development skills in the new year. These tutorials cover a range of topics, from foundatio
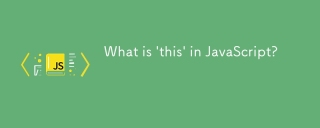
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
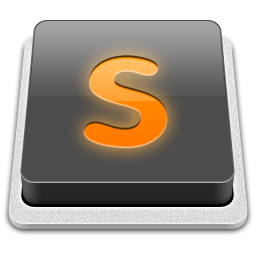
SublimeText3 Mac version
God-level code editing software (SublimeText3)
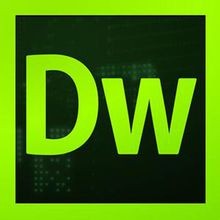
Dreamweaver CS6
Visual web development tools
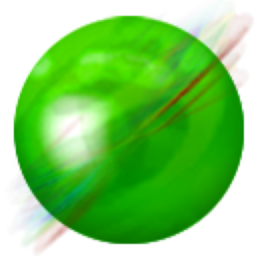
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
