


How to Build Unlimited Levels of Menus with PHP and MySQL: A Step-by-Step Guide?
Building Unlimited Levels of Menus with PHP and MySQL
In website development, menu navigation plays a crucial role in user experience. However, building menus that support unlimited levels of submenus can be challenging. Let's delve into a comprehensive approach to achieve this functionality using PHP and MySQL.
Database Structure and Menu System
The database structure you mentioned is a common approach to represent a hierarchical menu system. Each menu item has a parent field to establish the parent-child relationship. Parent ID 0 indicates top-level menu items.
Retrieving Top-Level Menus
Your existing PHP code for retrieving top-level menus is correct:
<code class="php">$list = $obj->childmenu($parentid); foreach($list as $menu) { echo '<li><a href="#">'.$name.'</a></li>'; }</code>
Identifying Submenu Existence and Display
Your query aims to identify if a menu item has child menus and display them within its list item. To achieve this, you need to modify your SQL query and PHP code.
Optimized SQL Query
Using a single SQL query, we can retrieve all menu items ordered by their parent ID and position:
<code class="sql">SELECT id, parent_id, title, link, position FROM menu_item ORDER BY parent_id, position;</code>
Refined PHP Code
The revised PHP code incorporates a stack to track the current menu level and ensures efficient handling of nested menus:
<code class="php">$items = $query_results; // Assuming $query_results contains the result of the SQL query $html = ''; $parent = 0; $parent_stack = array(); foreach ( $items as $item ) { if ( !empty($item['parent_id']) ) { $children[$item['parent_id']][] = $item; } } while ( ( $option = each( $children[$parent] ) ) || ( $parent > 0 ) ) { if ( !empty( $option ) ) { if ( !empty( $children[$option['value']['id']] ) ) { $html .= '<li>' . $option['value']['title'] . '<ul>'; array_push( $parent_stack, $parent ); $parent = $option['value']['id']; } else { $html .= '<li>' . $option['value']['title'] . '</li>'; } } else { $html .= '</ul>'; $parent = array_pop( $parent_stack ); } } echo $html;</li></code>
This updated code will effectively process the menu structure, create HTML markup for nested menus, and display them within their respective list items.
The above is the detailed content of How to Build Unlimited Levels of Menus with PHP and MySQL: A Step-by-Step Guide?. For more information, please follow other related articles on the PHP Chinese website!
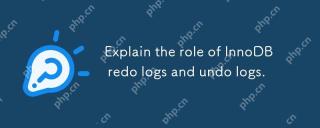
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
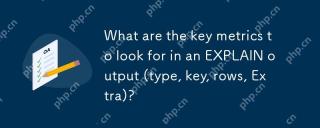
Key metrics for EXPLAIN commands include type, key, rows, and Extra. 1) The type reflects the access type of the query. The higher the value, the higher the efficiency, such as const is better than ALL. 2) The key displays the index used, and NULL indicates no index. 3) rows estimates the number of scanned rows, affecting query performance. 4) Extra provides additional information, such as Usingfilesort prompts that it needs to be optimized.
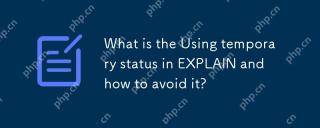
Usingtemporary indicates that the need to create temporary tables in MySQL queries, which are commonly found in ORDERBY using DISTINCT, GROUPBY, or non-indexed columns. You can avoid the occurrence of indexes and rewrite queries and improve query performance. Specifically, when Usingtemporary appears in EXPLAIN output, it means that MySQL needs to create temporary tables to handle queries. This usually occurs when: 1) deduplication or grouping when using DISTINCT or GROUPBY; 2) sort when ORDERBY contains non-index columns; 3) use complex subquery or join operations. Optimization methods include: 1) ORDERBY and GROUPB
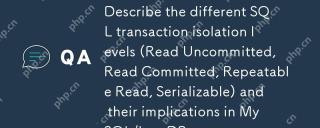
MySQL/InnoDB supports four transaction isolation levels: ReadUncommitted, ReadCommitted, RepeatableRead and Serializable. 1.ReadUncommitted allows reading of uncommitted data, which may cause dirty reading. 2. ReadCommitted avoids dirty reading, but non-repeatable reading may occur. 3.RepeatableRead is the default level, avoiding dirty reading and non-repeatable reading, but phantom reading may occur. 4. Serializable avoids all concurrency problems but reduces concurrency. Choosing the appropriate isolation level requires balancing data consistency and performance requirements.
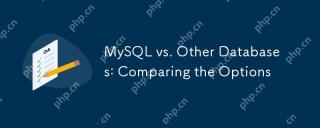
MySQL is suitable for web applications and content management systems and is popular for its open source, high performance and ease of use. 1) Compared with PostgreSQL, MySQL performs better in simple queries and high concurrent read operations. 2) Compared with Oracle, MySQL is more popular among small and medium-sized enterprises because of its open source and low cost. 3) Compared with Microsoft SQL Server, MySQL is more suitable for cross-platform applications. 4) Unlike MongoDB, MySQL is more suitable for structured data and transaction processing.
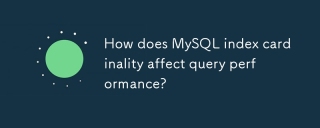
MySQL index cardinality has a significant impact on query performance: 1. High cardinality index can more effectively narrow the data range and improve query efficiency; 2. Low cardinality index may lead to full table scanning and reduce query performance; 3. In joint index, high cardinality sequences should be placed in front to optimize query.
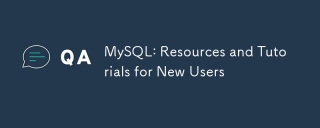
The MySQL learning path includes basic knowledge, core concepts, usage examples, and optimization techniques. 1) Understand basic concepts such as tables, rows, columns, and SQL queries. 2) Learn the definition, working principles and advantages of MySQL. 3) Master basic CRUD operations and advanced usage, such as indexes and stored procedures. 4) Familiar with common error debugging and performance optimization suggestions, such as rational use of indexes and optimization queries. Through these steps, you will have a full grasp of the use and optimization of MySQL.
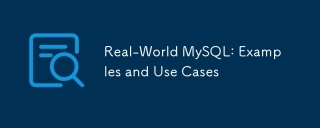
MySQL's real-world applications include basic database design and complex query optimization. 1) Basic usage: used to store and manage user data, such as inserting, querying, updating and deleting user information. 2) Advanced usage: Handle complex business logic, such as order and inventory management of e-commerce platforms. 3) Performance optimization: Improve performance by rationally using indexes, partition tables and query caches.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
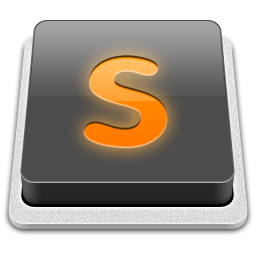
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
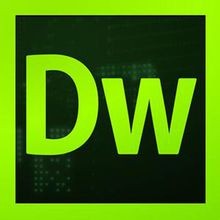
Dreamweaver CS6
Visual web development tools
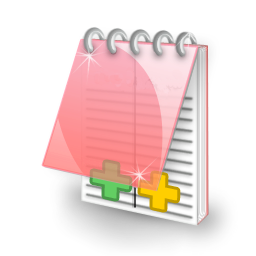
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function