


Why am I getting \'Cannot make a static reference to a non-static field/method\' in Java?
Understanding "Cannot Make a Static Reference to Non-Static Field/Method" in Java
In Java, encountering the error "Cannot make a static reference to the non-static field" or "Cannot make a static reference to the non-static method" indicates that certain actions are restricted due to the interaction between static and non-static elements in your code.
Cause of the Error
This error arises when you attempt to access instance (non-static) fields or methods within a static context, such as inside a static method. Instance variables are associated with specific objects of a class, while static variables and methods belong to the class itself and do not require object instances.
Solution: Create an Instance
To resolve the error, you need to create an instance of the class before accessing instance variables or invoking instance methods. This is because the instance variables and methods are not accessible directly from a static context.
For instance, in the provided code snippet, the static method main attempts to access instance variables r, cfr, and area, as well as instance methods c_cfr and c_area. To fix this, create an instance of the Cerchio class within the main method and then access the instance variables and methods through the object reference:
<code class="java">public static void main(String[] args) { Cerchio cerchio = new Cerchio(); cerchio.r = 5; cerchio.c_cfr(); cerchio.c_area(); System.out.println("The cir is: " + cerchio.cfr); System.out.println("The area is: " + cerchio.area); }</code>
Additional Notes
- Instance variables should generally be declared as private and accessed via getter and setter methods for encapsulation.
- Avoid directly accessing class variables; instead, use class constants or static methods to access them.
- Proper indentation and code organization can enhance readability and prevent such errors in the future.
The above is the detailed content of Why am I getting \'Cannot make a static reference to a non-static field/method\' in Java?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
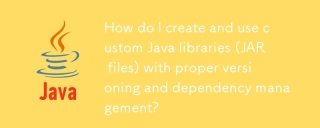
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
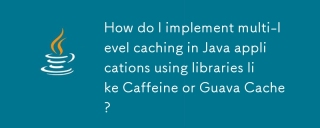
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
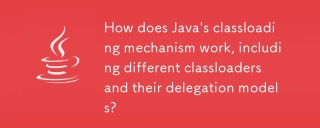
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
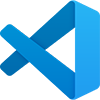
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
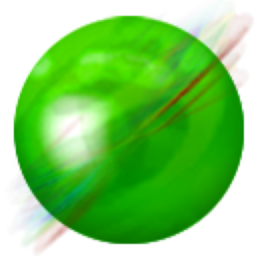
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
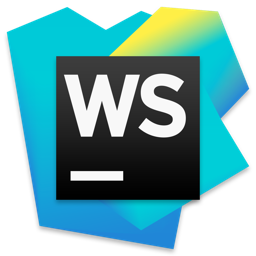
WebStorm Mac version
Useful JavaScript development tools